This article was published as a part of the Data Science Blogathon.
Introduction
is a type of NoSQL Database, that stores data in document format(bson or binary json format).
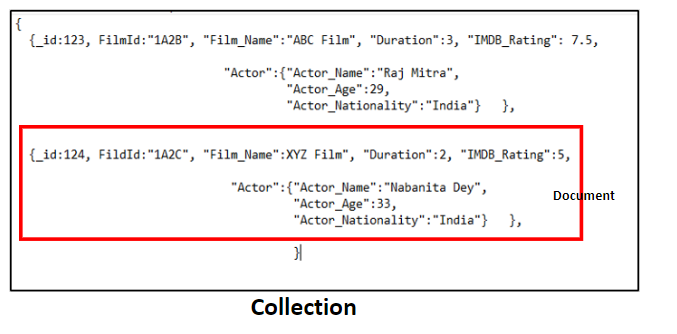
Its advantage over traditional SQL Databases includes the flexibility of schema-design, relaxation of its ACID properties and its distributed data storage capability thus performing better for storage of large-scale unstructured data.
There are different types of NoSQL databases; MongoDB falls under Document-based databases.
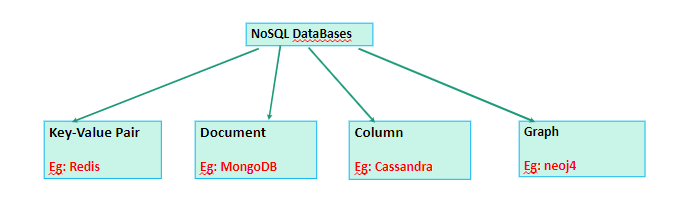
It’s called NoSQL because it is different from SQL databases in properties. The detailed difference and other salient features of MongoDB are given in my previous blog. I highly recommend reading that blog of mine first then coming here. The storage structure of MongoDB can be represented using the below hierarchical structure.
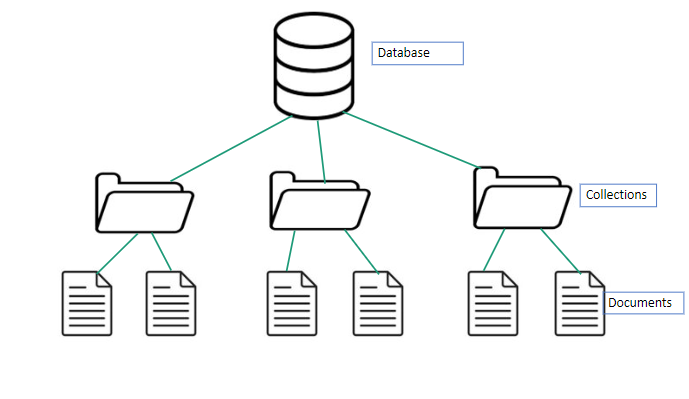
So a database contains single or multiple collections, each containing one or many documents. Collection can be thought of similar to Tables in RDBMS AND Documents as each record/tuple/row.
Important Points Regarding Syntax
Suppose we want to return all the documents where the name field is Aniket; the syntax would be:
db.collection.find({"name":"Aniket"})
Note here a few things, we have put the field name “name” and field value “Aniket” in double quotes. Fields are name-value pairs in MongoDB documents similar to columns in SQL tables. Putting field name in quotes is optional, we may or may not. But the value Aniket if it’s a string should always be in quotes. Also, we can use single quotes in place of double ones. But always advised following a single syntax to avoid confusion and mistakes.
So the below queries might also work and fetch same result.
db.collection.find({name:"Aniket"})
db.collection.find({"name":'Aniket'})
db.collection.find({'name':'Aniket'})
Also any operator/function we will use like $match, $lt, $regex has the same property and can be used with (“$regex”) or without ($regex) quotes.
Also, note that there is a reference number for each figure/screenshot I put. It indicates that you compare the resulting solution of a query with the screenshot of the given reference number to see the change.
Also, note another important point. For any operation like update or delete etc. there are two options UpdateOne/UpdateMany , DeleteOne/DeleteMany. Note that as the names indicate UpdateOne is used to update a single document and UpdateMany is used to update multiple documents at a time. The same goes for Delete.
CRUD Operations (BASIC)
CRUD stands for Create, Read, Update, Delete. So these operations when performed on the database, help us to view, search or modify data in our database. The syntax for the common CRUD operations which are generally useful for working with MongoDB is described below.
Operation1:
Operation- Create a database
Syntax- use database_name
Code:
use Sample_DataSet
So we created a database successfully. Notice the expression Sample_DataSet>
Operation2:
Operation- Create a collection within a database
Syntax- db.createCollection(name)
Code:
db.createCollection("Personal_Details")
Note: The above command should be typed when we are inside our desired database. In this case, we are inside Sample_Dataset database. We know that by the expression Sample_Dataset> as we can see in the above screenshot. It takes the name of the database we are currently inside.
Operation3:
Operation- View names of collections of a database.
Syntax- show collections
Code:
show collections
Note: Again just like the previous operation here also show collections must be typed when we are inside our desired database.
Operation4:
Operation- Delete a collection
Syntax- db.collection_name.drop()
Code:
db.Personal_Details.drop()
Note: When you do show collections again, you will see an empty result indicating our Personal_Details collection which we created earlier got deleted.
Operation5:
Operation- Insert a single document
Syntax- db.collection.insertOne({“key_a”:”value_a”, “key_b”:”value_b”})
Code:
db.Personal_Details.insertOne({"name":"Aniket", "age":19})
So as we can see, our record got inserted as it’s returning acknowledged: true, it also returned the id of our record. MongoDB attaches a unique id by default to every record we insert. That is referred to as ObjectId we can see in the above screenshot.
Operation6:
Operation- Insert multiple documents
Syntax- db.collection.insertMany([{“key1_a”:”value1_a”,”key1_b”:”value1_b”}, {“key2_a”:”value2_a”, “key2_b”:”value2_b”}])
Code:
db.Personal_Details.insertMany([{"name":"Anik","Age":77},{"name":"Banik", "Age":60}])
So we inserted two documents into our collection. (Check REF1 to see how our collection looks after insertion)
Operation7:
Operation- Query and return all documents of a collection
Syntax- db.collection.find()
Code:
db.Personal_Details.find()

REF:1
We returned all the three documents we inserted previously.
Operation8:
Operation- Rename a field name.
Syntax-
db.Personal_Details.updateMany({cond_field:cond_val},{$rename:{“Original_Field_Name”:”Renamed_Field_Name”}})
Note: Suppose you don’t want to update all documents, but a few on them based on some condition. For eg: you want to update the documents where the name is Aniket. So your cond_field is name and cond_val is Aniket
Code:
db.Personal_Details.updateMany({},{$rename:{"Age":"age"}})
REF: 2
Notice the screenshot of Operation7(REF 1) carefully. By mistake, the ‘Age’ field name is in caps for Anik and Banik but in lowercase in the case of Aniket. So to correct this mistake we used the OperatIon8 syntax to rename the Age field name for Anik and Banik to lowercase.(Compare with REF: 1 to see the change due to the query).
Operation9:
Operation- Update a single field value
Syntax-db.collection.updateOne({cond_field:cond_val},{$set:{“Original_Field_Value”:”Modified_Field_Value”})
Code:
db.Personal_Details.updateOne({name:"Anik"},{$set:{age:22}})

We successfully changed the age of Anik from 77 to 22. (Compare with REF: 2)
Operation10
Operation- Update multiple field value
Syntax-db.Personal_Details.updateMany({cond_field:cond_val}),{$set:{“Original_Fields_Value”:”Modified_Fields_Value”})
Code:
db.Personal_Details.updateMany({"name":/^A/},{$set:{age:20}})

We used a regex here. Note ^A denotes all strings which start with uppercase A. So through this query, we changed the age of all those individuals to 20 whose name begins with A. (Compare with REF: 3)
Operation11:
Operation- Delete a single document from a collection
Syntax- db.collection.deleteOne({cond_field:cond_value})
Code:
db.Personal_Details.deleteOne({"age":60})

Here we deleted the record/document of that individual whose age is 60 i.e Banik‘s. (Compare with REF: 4)
Operation12:
Operation- Delete multiple documents from a collection
Syntax- db.collection.deleteMany({cond_field:cond_value})
Code:
db.Personal_Details.deleteMany({"name":/^Anik/})

So we deleted all the documents for which the name starts with Anik. Now our collection becomes empty since we already deleted Banik‘s record in Operation11. So when we try to use .find(), we get an empty result. (Compare with REF: 5)
(We will again reinstate the deleted records so that we can use them for other operations.)
Operation13:
Operation- Drop a whole collection
Syntax- db.collection.drop()
Code:
db.Personal_Details.drop()

We deleted our Personal_Details collection successfully, which is why when we are doing show collections it is returning nothing.
Operation14:
Operation- Delete all documents of a collection
Syntax- db.collection.deleteMany()
Code:
db.Personal_Details.deleteMany({})


As seen from the above two pics, we have deleted all the 3 documents of Personal_Details and made it empty. (Compare with REF 7)
Operation15:
Operation- Drop whole database
Syntax- db.dropDatabase()
Code:
db.dropDatabase()

So we dropped our Sample_DataSet database. Remember to use this command inside the database you want to delete.
CRUD Operations (ADVANCED)
Operation1:
Operation- Use less than condition to find documents.
Syntax- db.collection.find({field:{$lt:value}})
Code:
db.Personal_Details.find({age:{$lt:65}})

Here we found out all individuals whose ages are less than 65. (Compare with REF 7)
Less than equals(<=) | Greater than(>) | Greater than equals(>=) | Not equals(!=) |
$lte | $gt | $gte | $ne |
The above table denotes the common filter operations, the overall syntax is similar to $lt of Operation1, just replace $lt with your desired symbol. (Compare with REF 7)
Operation2:
Operation- Find documents using OR condition
Syntax- db.collection.find({$or:[{cond_field1:cond_val1},{cond_field2:cond_val2}]})
Code:
db.Personal_Details.find({$or:[{name:"Anik"},{age:{$gt:50}}]})

In the above code, we tried to find out all records which either have name as Anik or age greater than 50 or both. (Compare with REF 7)
Note similar to OR, we can also use AND operations in MongoDB search. The syntax is simple. Replace $or with $and . So now it will only return those records where both conditions satisfies i.e, name is Anik and age greater than 50.
Operation3:
Operation- Find documents based on the combination of AND and OR
Syntax- It varies according to condition. For example, suppose in our case we want to find out the records of all individuals who have their name as Aniket and age lesser than equals 20 or any individuals whose age greater than 70.
Code:
db.Personal_Details.find({$or:[{$and:[{name:"Aniket"},{age:{$lte:20}}]},{age:{$gt:70}}]})

So we returned Aniket‘s record whose name is Aniket and age lesser than equals 20 and also Anik‘s record whose age is greater than 70. (Compare with REF 7)
Operation4:
Operation- Return a document/record based on a filter on the values of a nested .json field(field with another json document inside.)
For eg: suppose we add the address details of Aniket. So our collection now will look like this.

REF 8
Note that here there is another .json like field address is added only in the case of Aniket which contains two more fields nested in it- state and country.
Syntax- db.collection.find({“field.subfield”:val})
Code:
db.Personal_Details.find({"address.country":"India"})

Here we found our records of all individuals whose country subfield in the address field is India. (Compare with REF 8)
Operation5:
Operation- Add an extra field only to a single/few documents of the collection, not all.
Syntax-db.collection.updateOne/updateMany({cond_field:cond_val},{$set:{new_field_name:new_field_val}})
Code:
db.Personal_Details.updateOne({name:"Aniket"},{address:{state:"Maharashtra", country:"India"}})

So we added a new field called address which has state and country subfields. Note that it is added to only Aniket’s record. (Compare with REF 7)
(The above new schema only we used in Operation4 if you notice. !!)
Operation6:
Operation- Sort the results obtained from a query.
Syntax- db.collection.find({cond_field:cond_val}).sort({field:1})
Note- 1 -> sort result in ascending order.
-1 -> sort result in descending order.
Code:
db.Personal_Details.find().sort({age:-1})

So we sorted our results in descending order of age. (Compare with REF 8)
Operation7:
Operation- Query an array field.
Syntax- db.collection.find({arr_field:[a,b,c,…..]})
We are going to query on the following data:

REF 9
Code:
db.Personal_Details.find({scores:[57,78]})

So we obtained the record with scores field containing the exactly same array [57,78]. Notice that Banik also contains these two scores(both 57 and 78) but two other additional scores 87 and 44 also thus it’s not returned. Aniket, on the other hand, contains only one out of the two values queries i.e only 78 so it’s also not returned. (Compare with REF 9)
Operation8:
Operation- Return record if the field contains atleast one of the objects/values passed in the query array.
Syntax- db.collection.find({field:{$in:[a,b,c,…..]}})
Code:
db.Personal_Details.find({scores:{$in:[57,78]}})

So we can see all the 3 records returns as they contain atleast one of 57 or 78. (Compare with REF 9)
Operation9:
Operation- Return the records based on equality condition using $elemMatch from an array of embedded docs.
Below you can see an array of embedded docs

REF 10
Syntax- db.collection.find({$elemMatch:{field:value}})
Code:
db.Personal_Details.find({"Education":{$elemMatch:{"College":"XYZ College"}}})

We searched the College named XYZ college using $elemMatch and got our result. (Compare with REF 10)
Operation10:
Operation- Return only the required fields from our result, not all the fields.
Syntax- db.collection.find({field:val},{_id:0,field_name:1})
To return only the field we want we have to do field_name:1, where 1 means return it and 0 means don’t return it. Remember, if you do not mention anything, the _id field will always be returned. If you don’t need it then do _id:0
Code:;
db.Personal_Details.find({},{_id:0,name:1})

So we returned only name field from our query. (Compare with REF 10)
Operation11:
Operation- Return all documents where a particular field name exists
Syntax- db.collection.find({field:{$exists:true}})
if you need to return the documents where the field is not present use $exists:false
Code:
db.Personal_Details.find({"Education":{"$exists":true}})

So we only returned the documents where Education field is present. (Compare with REF 10)
CRUD Operations (MORE QUERIES !!! 😊)
Operation1:
Operation- Find all the documents with arrays matching a list of elements.
Syntax- db.collection.find({field:[“A”,”B”,…..]})
Code:
db.Personal_Details.find({scores:{$all:[57,78]}})

As we can see our query returned only the records where scores contain both 57,78 and maybe some more values. (Compare with REF 10)
Notice the difference btw $in and $all operators when we use them with arrays. We discussed $in before.
Operation2:
Operation- Return records with arrays with a specific size(no. of elements) only.
Syntax- db.collection.find({field:{$size:num}})
Code:
db.Personal_Details.find({scores:{$size:4}})

We only returned the records with scores size 4. (Compare with REF 10)
Operation3:
Operation- Return the first few elements of the array field of a document.
Syntax- db.collection.find({cond_field:cond_val},{skills:{$slice:num}})
Code:
db.Personal_Details.find({},{scores:{$slice:1}})
We returned one value in scores array for every record since we passed parameter $slice:1. (Compare with REF 10)
Operation4:
Operation- Use NOR operator to choose records exactly opposite to the given conditions.
NOR is as we all know OR+NOT. So the records are fetched by applying OR condition, we will leave and return those which are not fetched by OR.
Syntax- db.collection.find({$nor:[{cond_field1:cond_val1},{cond_field2:cond_val2}]})
Code:
db.Personal_Details.find({$nor:[{age:{$lt:20}},{age:{$gt:75}}]})
So as expected our query returned exactly the opposite of our conditions i.e, not lesser than 20 or not greater than 75, or both. (Compare with REF 10)
Operation5:
Operation- Return all records except matching the given condition using not equals operator.
Syntax- db.collection.find({cond_field:{$ne:cond_val}})
Code:
db.Personal_Details.find({name:{$ne:"Aniket"}})
So we can see that we returned the records which are opposite to the given condition i.e, we returned records where name is not Aniket. (Compare with REF 10)
Operation6:
Operation- Use regex to return records that match a particular pattern.
Syntax- db.collection.find({cond_field:{$regex:regex_pattern}})
Code:
db.Personal_Details.find({"name":{$regex:/^A/}})
We found all the records whose name starts with A. (Compare with REF 10)
Operation7:
Operation- Increment the value of a field by using $inc operator.
Syntax- db.collection.find({cond_field:cond_val},{$inc:{field_to_inc:inc_val}})
Code:
db.Personal_Details.find({name:"Anik"},{$inc:{"age":2}})
REF 11
As we can see, Anik‘s age increased by 2 i.e, from 77 to 79. (Compare with REF 10)
Operation8:
Operation- Update the value of a field by multiplying it with a specified number.
Syntax- db.collection.find({cond_field:cond_val},{$mul:{field_to_mul:mul_factor}})
Code:
db.Personal_Details.updateOne({"name":"Banik"},{$mul:{age:1.1}})
REF 12
As we can see Banik‘s age got multiplied with 1.1 i.e, changed from 60 to 66. (Compare with REF 11)
Operation9:
Operation- Remove the first element from an array field.
Syntax-
db.collection.updateOne/updateMany({cond_field:cond_val},{$pop:{array_field:num_of_array_to_remove}})
Note: num_to_array_to_remove=1 indicates we remove the last element of array, -1 indicates first element to remove
Code:
db.Personal_Details.updateOne({name:"Banik"},{$pop:{scores:1}})
REF 13
We removed the last element of scores field of Banik‘s record. (Compare with REF12)
Operation10:
Operation- Remove a number of elements from an array based on a condition.
Syntax- db.collection.updateOne/updateMany({cond_field:cond_val},{$pull:{array_field:cond}})
Code:
db.Personal_Details.updateOne({"name":"Banik"},{$pull:{scores:{$gt:60}}})
REF 14
We pulled out/removed all the values of scores field of Banik‘s record which are greater than 60. (Compare with REF13)
Operation11:
Operation- Add an element to an array field.
Syntax- db.collection.updateOne/updateMany({cond_field:cond_val},{$push:{array_field:value}})
Code:
db.Personal_Details.updateOne({name:"Banik"},{$push:{scores:60}})

We pushed 60 to scores field of Banik‘s. (Refer to REF 14)
Conclusion
We seemed to cover a huge number of queries. Let’s stop for now. There are some more advanced queries but already the blog has become very big, so I will discuss more queries in the rest of the blog.
There are some points that should be remembered while writing the syntaxes for CRUD Operations:
1. The performance of a query operation can be improved by doing proper Indexing.
2. Complex operations can be performed by combining one or more of these operations together. You may have noticed I have already performed such an operation by combining $AND & $OR operators.
3. Proper Data Modelling helps in faster query retrieval, especially in the case of aggregation queries which we will discuss in some other blogs.
The media shown in this article is not owned by Analytics Vidhya and is used at the Author’s discretion.
Related
- SEO Powered Content & PR Distribution. Get Amplified Today.
- Platoblockchain. Web3 Metaverse Intelligence. Knowledge Amplified. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2022/12/crud-operations-in-mongodb/