We know that various forms of written communication, like social media and emails, generate vast volumes of unstructured textual data. This data contains valuable insights and information. However, manually extracting relevant insights from large amounts of raw text is highly labor-intensive and time-consuming. Text mining addresses this challenge. Using computer techniques it refers to automatically analyzing and transforming unstructured text data to discover patterns, trends, and essential information. Computers have the ability to process text written in human languages thanks to text mining. To find, extract, and measure relevant information from large text collections, it uses natural language processing techniques.
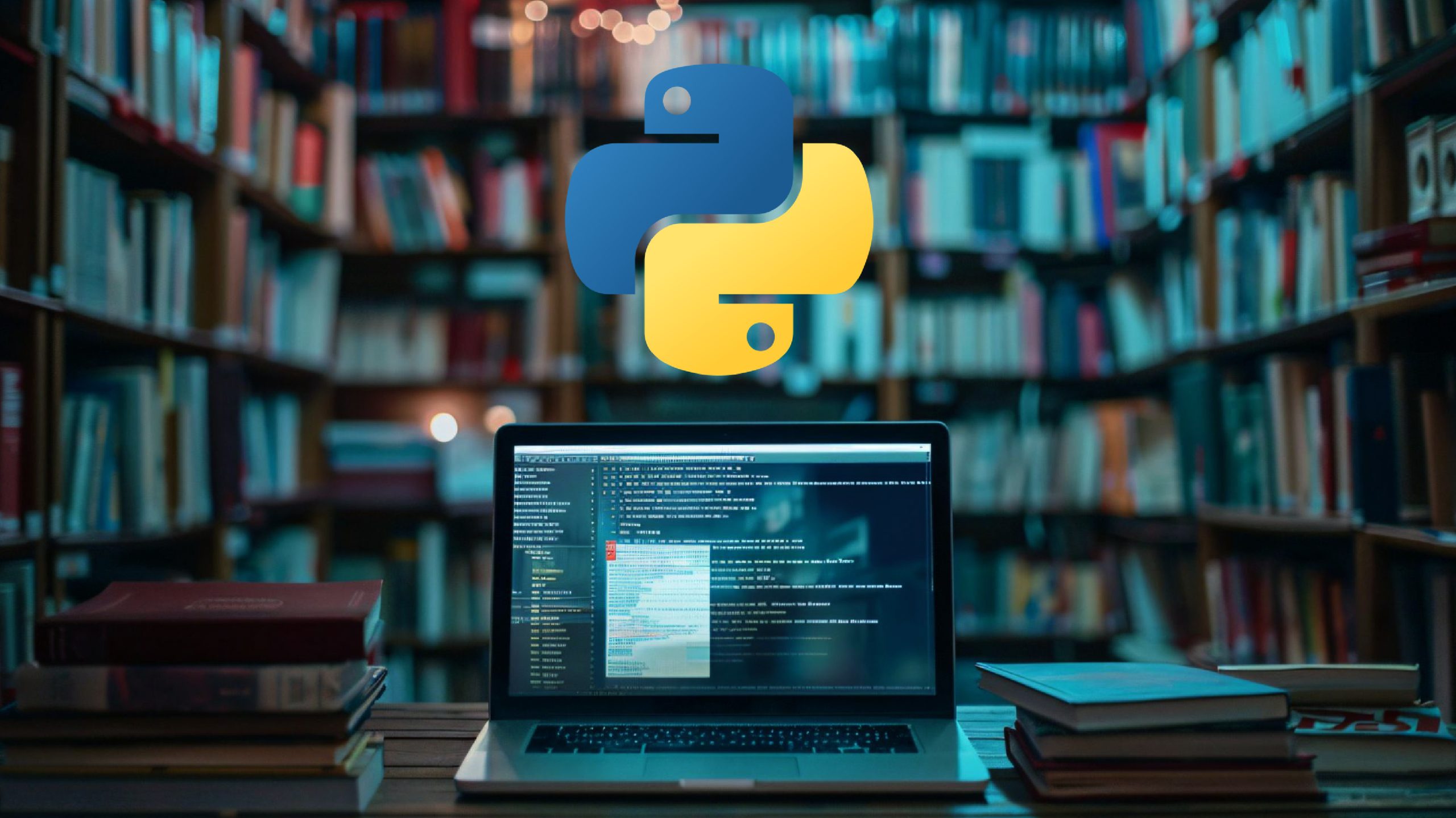
Overview
- Understand text mining and its importance in various fields.
- Learn basic text mining techniques like tokenization, stop words removal and POS tagging.
- Explore real-world applications of text mining in sentiment analysis and named entity recognition.
Table of contents
Importance of Text Mining in the Modern World
Text mining is important in many areas. It helps businesses understand what customers feel and improve marketing. In healthcare, it is used to look at patient records and research papers. It also helps the police by checking legal documents and social media for threats. Text mining is key for pulling useful information from text in different industries.
Understanding Natural Language Processing
Natural Language Processing is a type of artificial intelligence. It helps computers understand and use human language to communicate with people. NLP allows computers to interpret and respond to what we say in a way that makes sense.
Key Concepts in NLP
- Stemming and Lemmatization: Reduce words to their basic form.
- Stop Words: Remove common words like “the,” “is,” and “at” that don’t add much meaning.
- Part-of-Speech Tagging: Assign parts of speech, like nouns, verbs, and adjectives, to each word.
- Named Entity Recognition (NER): Identify proper names in text, such as people, organizations, and locations.
Getting Started with Text Mining in Python
Let us now look into the steps with which we can get started with text mining in Python.
Step1: Setting Up the Environment
To start text mining in Python, you need a suitable environment. Python provides various libraries that simplify text mining tasks.
Make sure you have Python installed. You can download it from python.org.
Set Up a Virtual Environment by typing the following code. It’s a good practice to create a virtual environment. This keeps your project dependencies isolated.
python -m venv textmining_env
source textmining_env/bin/activate # On Windows use `textmining_envScriptsactivate`
Step2: Installing Necessary Libraries
Python has several libraries for text mining. Here are the essential ones:
- NLTK (Natural Language Toolkit): A powerful library for NLP.
pip install nltk
- Pandas: For data manipulation and analysis.
pip install pandas
- NumPy: For numerical computations.
pip install numpy
With these libraries, you are ready to start text mining in Python.
Basic Terminologies in NLP
Let us explore basic terminologies in NLP.
Tokenization
Tokenization is the first step in NLP. It involves breaking down text into smaller units called tokens, usually words or phrases. This process is essential for text analysis because it helps computers understand and process the text.
Example Code and Output:
import nltk
from nltk.tokenize import word_tokenize
# Download the punkt tokenizer model
nltk.download('punkt')
# Sample text
text = "In Brazil, they drive on the right-hand side of the road."
# Tokenize the text
tokens = word_tokenize(text)
print(tokens)
Output:
['In', 'Brazil', ',', 'they', 'drive', 'on', 'the', 'right-hand', 'side', 'of', 'the', 'road', '.']
Stemming
Stemming reduces words to their root form. It removes suffixes to produce the stem of a word. There are two common types of stemmers: Porter and Lancaster.
- Porter Stemmer: Less aggressive and widely used.
- Lancaster Stemmer: More aggressive, sometimes removing more than necessary.
Example Code and Output:
from nltk.stem import PorterStemmer, LancasterStemmer
# Sample words
words = ["waited", "waiting", "waits"]
# Porter Stemmer
porter = PorterStemmer()
for word in words:
print(f"{word}: {porter.stem(word)}")
# Lancaster Stemmer
lancaster = LancasterStemmer()
for word in words:
print(f"{word}: {lancaster.stem(word)}")
Output:
waited: wait
waiting: wait
waits: wait
waited: wait
waiting: wait
waits: wait
Lemmatization
Lemmatization is similar to stemming but considers the context. It converts words to their base or dictionary form. Unlike stemming, lemmatization ensures that the base form is a meaningful word.
Example Code and Output:
import nltk
from nltk.stem import WordNetLemmatizer
# Download the wordnet corpus
nltk.download('wordnet')
# Sample words
words = ["rocks", "corpora"]
# Lemmatizer
lemmatizer = WordNetLemmatizer()
for word in words:
print(f"{word}: {lemmatizer.lemmatize(word)}")
Output:
rocks: rock
corpora: corpus
Stop Words
Stop words are common words that add little value to text analysis. Words like “the”, “is”, and “at” are considered stop words. Removing them helps focus on the important words in the text.
Example Code and Output:
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
# Sample text
text = "Cristiano Ronaldo was born on February 5, 1985, in Funchal, Madeira, Portugal."
# Tokenize the text
tokens = word_tokenize(text.lower())
# Remove stop words
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
# Download the stopwords corpus
nltk.download('stopwords')
# Remove stop words
stop_words = set(stopwords.words('english'))
filtered_tokens = [word for word in tokens if word not in stop_words]
print(filtered_tokens)
Output:
['cristiano', 'ronaldo', 'born', 'february', '5', ',', '1985', ',', 'funchal', ',', 'madeira', ',', 'portugal', '.']
Advanced NLP Techniques
Let us explore advanced NLP techniques.
Part of Speech Tagging (POS)
Part of Speech Tagging means marking each word in a text as a noun, verb, adjective, or adverb. It’s key for understanding how sentences are built. This helps break down sentences and see how words connect, which is important for tasks like recognizing names, understanding emotions, and translating between languages.
Example Code and Output:
import nltk
from nltk.tokenize import word_tokenize
from nltk import ne_chunk
# Sample text
text = "Google's CEO Sundar Pichai introduced the new Pixel at Minnesota Roi Centre Event."
# Tokenize the text
tokens = word_tokenize(text)
# POS tagging
pos_tags = nltk.pos_tag(tokens)
# NER
ner_tags = ne_chunk(pos_tags)
print(ner_tags)
Output:
(S
(GPE Google/NNP)
's/POS
(ORGANIZATION CEO/NNP Sundar/NNP Pichai/NNP)
introduced/VBD
the/DT
new/JJ
Pixel/NNP
at/IN
(ORGANIZATION Minnesota/NNP Roi/NNP Centre/NNP)
Event/NNP
./.)
Chunking
Chunking groups small units, like words, into bigger, meaningful units, like phrases. In NLP, chunking finds phrases in sentences, such as noun or verb phrases. This helps understand sentences better than just looking at words. It’s important for analyzing sentence structure and pulling out information.
Example Code and Output:
import nltk
from nltk.tokenize import word_tokenize
# Sample text
text = "We saw the yellow dog."
# Tokenize the text
tokens = word_tokenize(text)
# POS tagging
pos_tags = nltk.pos_tag(tokens)
# Chunking
grammar = "NP: {<DT>?<JJ>*<NN>}"
chunk_parser = nltk.RegexpParser(grammar)
tree = chunk_parser.parse(pos_tags)
print(tree)
Output:
(S (NP We/PRP) saw/VBD (NP the/DT yellow/JJ dog/NN) ./.)
Chunking helps in extracting meaningful phrases from text, which can be used in various NLP tasks such as parsing, information retrieval, and question answering.
Practical Examples of Text Mining
Let us now explore practical examples of text mining.
Sentiment Analysis
Sentiment analysis identifies emotions in text, like whether it’s positive, negative, or neutral. It helps understand people’s feelings. Businesses use it to learn customer opinions, monitor their reputation, and improve products. It’s commonly used to track social media, analyze customer feedback, and conduct market research.
Text Classification
Text classification is about sorting text into set categories. It’s used a lot in finding spam, analyzing feelings, and grouping topics. By automatically tagging text, businesses can better organize and handle lots of information.
Named Entity Extraction finds and sorts specific things in text, like names of people, places, organizations, and dates. It is used to get information, pull out important facts, and improve search engines. NER turns messy text into organized data by identifying key elements.
Text mining is used in many areas:
- Customer Service: It helps automatically analyze customer feedback to make service better.
- Healthcare: It pulls out important details from clinical notes and research papers to help in medical studies.
- Finance: It looks at financial reports and news articles to help make smarter investment choices.
- Legal: It speeds up the review of legal documents to find important information quickly.
Conclusion
Text mining in Python cleans up messy text and finds useful insights. It uses techniques like breaking text into words (tokenization), simplifying words (stemming and lemmatization), and labeling parts of speech (POS tagging). Advanced steps like identifying names (named entity recognition) and grouping words (chunking) improve data extraction. Practical uses include analyzing emotions (sentiment analysis) and sorting texts (text classification). Case studies in e-commerce, healthcare, finance, and legal show how text mining leads to smarter decisions and new ideas. As text mining evolves, it becomes essential in today’s digital world.
Frequently Asked Questions
A. Text mining is the process of utilizing computational techniques to extract meaningful patterns and trends from large volumes of unstructured textual data.
A. Text mining plays a crucial role in unlocking valuable insights that are often embedded within vast amounts of textual information.
A. Text mining finds applications in various domains, including sentiment analysis of customer reviews and named entity recognition within legal documents.
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/06/text-mining-in-python/