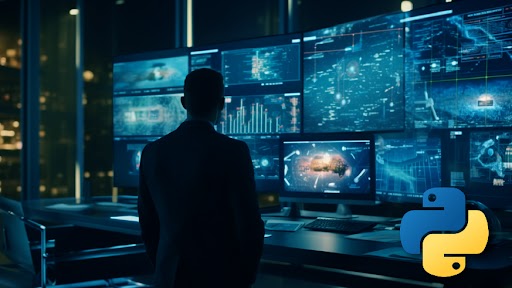
Introduction
Python is a powerful and versatile programming language with many built-in functions. One such function is reduce(), a tool for performing functional computations. It helps reduce a list of values to a single result. By applying a function to the iterable’s elements, reduce() returns a single cumulative value. This reduce() function is part of Python’s functools module and is widely used in various applications.
Overview
- Learn about the reduce() function in Python and how it works.
- Discover the syntax and parameters of reduce().
- Explore the importance and use cases of reduce() through examples.
Table of contents
What is reduce() Function in Python?
The reduce() function in Python performs cumulative operations on iterables. It takes two main arguments: a function and an iterable. By applying the function cumulatively to the iterable’s elements, reduce() reduces them to a single value. This makes it particularly useful for tasks such as summing numbers or finding the product of elements in a list.
How Does reduce() Work?
The reduce() function starts with the first two elements of an iterable, applies the function to them, then uses the result with the next element. This process continues until all elements are processed, resulting in a single cumulative value.
Syntax and Parameters
To use the reduce() function, import it from the functools module. The basic syntax is:
from functools import reduce
result = reduce(function, iterable[, initializer]
Explanation of Parameters:
- function: The function to apply to the elements of the iterable. It must take two arguments.
- iterable: The iterable whose elements you want to reduce. It can be a list, tuple, or any other iterable.
- initializer (optional): The starting value. It is used as the first argument in the first function call if provided.
Also Read: What are Functions in Python and How to Create Them?
Application of reduce() With an Initializer
from functools import reduce
numbers = [1, 2, 3, 4]
sum_result = reduce(lambda x, y: x + y, numbers, 0)
print(sum_result) # Output: 10
In this example, the initializer 0 ensures the function handles empty lists correctly.
By understanding the syntax and parameters of reduce(), you can leverage its power to simplify many common data processing tasks in Python.
Importance and Use Cases of reduce() Function in Python
The reduce() function is precious when processing data iteratively, avoiding explicit loops and making the code more readable and concise. Some common use cases include:
- Summing numbers in a list: Quickly add up all elements.
- Multiplying elements of an iterable: Calculate the product of elements.
- Concatenating strings: Join multiple strings into one.
- Finding the maximum or minimum value: Determine the largest or smallest element in a sequence.
Examples of Using reduce() Function in Python
Here are some examples of using reduce() function in Python:
Summing Elements in a List
The most common use case for reduce() is summing elements in a list. Here’s how you can do it:
from functools import reduce
numbers = [1, 2, 3, 4, 5]
sum_result = reduce(lambda x, y: x + y, numbers)
print(sum_result) # Output: 15
The reduce() function takes a lambda function that adds two numbers and applies it to each pair of elements in the list, resulting in the total sum.
Finding the Product of Elements
You can also use reduce() to find the product of all elements in a list:
from functools import reduce
numbers = [1, 2, 3, 4, 5]
product_result = reduce(lambda x, y: x * y, numbers)
print(product_result) # Output: 120
Here, the lambda function lambda x, y: x * y multiplies each pair of numbers, giving the product of all elements in the list.
Finding the Maximum Element in a List
To find the maximum element in a list using reduce(), you can use the following code:
from functools import reduce
numbers = [4, 6, 8, 2, 9, 3]
max_result = reduce(lambda x, y: x if x > y else y, numbers)
print(max_result) # Output: 9
The lambda function lambda x, y: x if x > y else y compares each pair of elements and returns the greater of the two, ultimately finding the maximum value in the list.
Advanced Uses of reduce() Function in Python
Let us now look at some advanced use cases of this Python Function:
Using reduce() with Operator Functions
Python’s operator module provides built-in functions for many arithmetic and logical operations, which are useful with reduce() to create cleaner code.
Example using operator.add to sum a list:
from functools import reduce
import operator
numbers = [1, 2, 3, 4, 5]
sum_result = reduce(operator.add, numbers)
print(sum_result) # Output: 15
Using operator.mul to find the product of a list:
from functools import reduce
import operator
numbers = [1, 2, 3, 4, 5]
product_result = reduce(operator.mul, numbers)
print(product_result) # Output: 120
Operator functions make the code more readable and efficient since they are optimized for performance.
Comparison with Other Functional Programming Concepts
In functional programming, reduce() is often compared with map() and filter(). While map() applies a function to each element of an iterable and returns a list of results, reduce() combines elements using a function to produce a single value. filter(), conversely, selects elements from an iterable based on a condition.
Here’s a quick comparison:
- map(): Transforms each element in the iterable.
- filter(): Selects elements that meet a condition.
- reduce(): Combines elements into a single cumulative result.
Each function serves a unique purpose in functional programming and can be combined to perform more complex operations.
Common Pitfalls and Best Practices
Let us look at some common pitfalls and best practices:
Handling Empty Iterables
One common pitfall when using the reduce() function is handling empty iterables. Passing an empty iterable to reduce() without an initializer raises a TypeError because there’s no initial value to start the reduction process. To avoid this, always provide an initializer when the iterable might be empty.
Example: Handling empty iterable with an initializer
from functools import reduce
numbers = []
sum_result = reduce(lambda x, y: x + y, numbers, 0)
print(sum_result) # Output: 0
In this example, the initializer 0 ensures that reduce() returns a valid result even if the list is empty.
Choosing reduce() Over Other Built-in Functions
While reduce() is powerful, it’s not always the best choice. Python provides several built-in functions that are more readable and often more efficient for specific tasks.
- Use sum() for summing elements: Instead of using reduce() to sum elements, use the built-in sum() function.
- Use max() and min() for finding extremes: Instead of reduce (), use max() and min() to find the maximum or minimum value.
Performance Considerations
Efficiency of reduce() Compared to Loops
The reduce() function can be more efficient than explicit loops because it is implemented in C, which can offer performance benefits. However, this advantage is often marginal and depends on the complexity of the function being applied.
Performance Benefits of Using Built-in Functions
Built-in functions like sum(), min(), and max() are highly optimized for performance. They are implemented in C and can perform operations faster than equivalent Python code using reduce().
Conclusion
In conclusion, the reduce() function is a versatile and powerful tool in Python’s functools module. It enables you to perform cumulative computations on iterables efficiently, simplifying tasks such as summing numbers, finding products, and identifying maximum values. Additionally, consider using built-in functions like sum(), max(), and min() for simpler tasks. Alternatives like the accumulate() function from the itertools module and traditional loops or list comprehensions can also be effective depending on the situation. By understanding when and how to use reduce(), you can write more efficient, readable, and elegant Python code.
Seize the opportunity to enhance your skills and propel your career forward. Join Analytics Vidhya’s free course on Introduction to Python!
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/06/reduce-function-in-python/