Introduction
In mathematics, we use exponents or powers to demonstrate the repeated multiplication of a number by itself. Python offers multiple ways to accomplish exponentiation, providing simplicity and adaptability for a range of use situations. Python provides powerful tools to perform a wide range of tasks, from simple math operations to intricate scientific calculations. You can access these capabilities through the ** operator, the math.pow() function from the math module, or the built-in pow() function. In this article we will look into the detail about exponents in Python. We will also learn about advanced exponentiation techniques, optimizations and best practices and the common pitfall also.
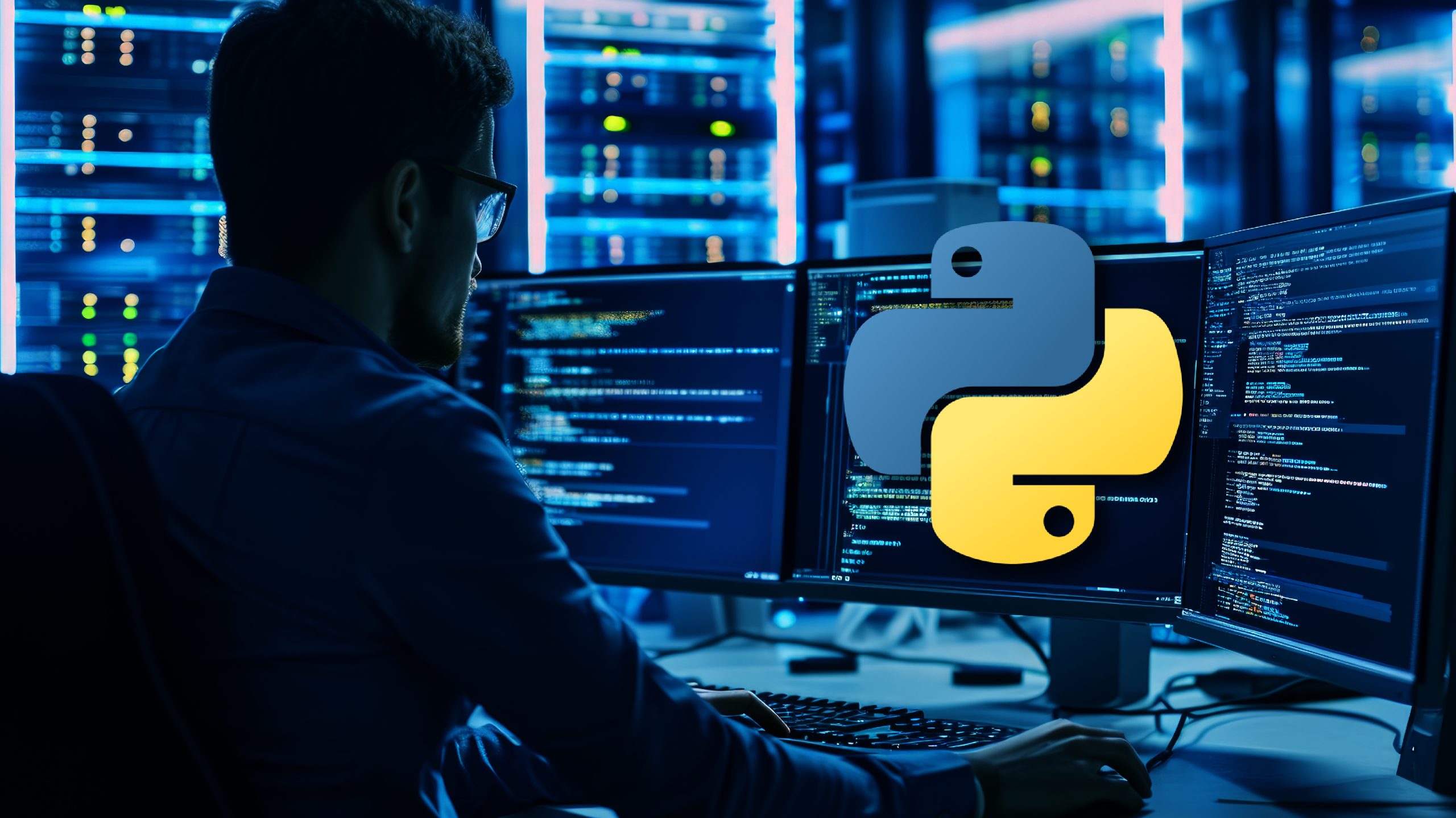
Table of contents
Basic Methods to Calculate Exponents in Python
In mathematics, we fundamentally use exponents, also known as powers, and we can compute them using various methods in Python. The **
operator, the pow() function, and math.pow() from the math module are commonly used to perform exponentiation, providing flexibility and precision in different scenarios.
Using the **
Operator
The **
operator is the most straightforward way to perform exponentiation in Python. It works for both integers and floating-point numbers.
base = 3
exponent = 4
result = base ** exponent
print(f"{base} to the power of {exponent} is {result}")
# Output: 3 to the power of 4 is 81
Using the pow() Function
You can use the built-in pow() function for exponentiation, and it provides an additional third parameter for modular arithmetic.
base = 5
exponent = 3
result = pow(base, exponent)
print(f"{base} to the power of {exponent} is {result}")
# Output: 5 to the power of 3 is 125
Using the math.pow() Function
The math.pow() function from the math module is specifically for floating-point exponentiation and returns a float.
import math
base = 2
exponent = 8
result = math.pow(base, exponent)
print(f"{base} to the power of {exponent} is {result}")
# Output: 2 to the power of 8 is 256.0
Advanced Exponentiation Techniques
Python advanced exponentiation techniques include handling fractional and negative exponents, effectively handling huge computations, and investigating exponentiation of complex numbers. These methods make use of Python’s adaptability and built-in functions to manage a variety of mathematical tasks.
Negative and Fractional Exponents
Python supports negative and fractional exponents, allowing for calculations of reciprocals and roots.
base = 16
negative_exponent = -2
fractional_exponent = 0.5
result_negative = base ** negative_exponent
result_fractional = base ** fractional_exponent
print(f"{base} to the power of {negative_exponent} is {result_negative}")
print(f"{base} to the power of {fractional_exponent} is {result_fractional}")
# Output: 16 to the power of -2 is 0.00390625
# 16 to the power of 0.5 is 4.0
Handling Large Exponents
Large exponent calculations can result in very large numbers. Python’s integer type can handle arbitrarily large values, but you should consider performance and memory usage.
large_base = 2
large_exponent = 1000
result = large_base ** large_exponent
print(f"{large_base} to the power of {large_exponent} has {len(str(result))} digits")
#output: 2 to the power of 1000 has 302 digits
Complex Number Exponentiation
Using the cmath module, Python can handle complex number exponentiation.
import cmath
base = complex(1, 2)
exponent = 3
result = base ** exponent
print(f"({base}) to the power of {exponent} is {result}")
# Output: ((1+2j)) to the power of 3 is (-11+2j)
Practical Applications of Exponents
People use Python, a flexible program, in computer science, physics, economics, finance, and other fields for scientific and computational applications involving exponents. With the use of its strong tools, one may compute compound interest, simulate growth and decay processes, and resolve challenging mathematical issues.
Growth Calculations (e.g., compound interest)
When computing compound interest, where the amount increases exponentially over time, exponents play a critical role.
principal = 1000
rate = 0.05
times_compounded = 4
years = 5
amount = principal * (1 + rate/times_compounded) ** (times_compounded * years)
print(f"Amount after {years} years: {amount}")
# Output: Amount after 5 years: 1283.36
Scientific Calculations (e.g., exponential decay)
Exponential decay describes processes that decrease rapidly at first and then level off. It’s commonly used in science and engineering.
initial_amount = 100
decay_constant = 0.1
time = 10
remaining_amount = initial_amount * math.exp(-decay_constant * time)
print(f"Remaining amount after {time} units of time: {remaining_amount}")
# Output: Remaining amount after 10 units of time: 36.79
Computer Graphics and Animations
In computer graphics, exponential functions are used to simulate a variety of natural occurrences and animations.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 10, 100)
y = np.exp(x)
plt.plot(x, y)
plt.title('Exponential Growth')
plt.xlabel('x')
plt.ylabel('exp(x)')
plt.show()
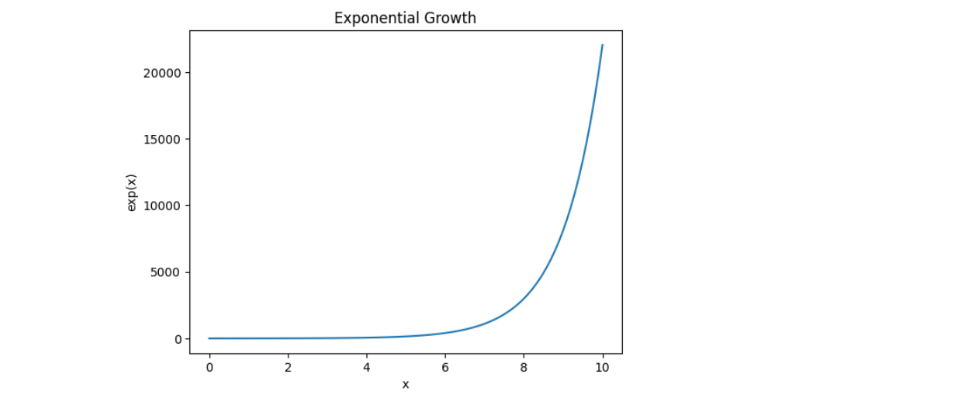
Optimizations and Best Practices
Consider optimizing best practices and utilizing these pointers and strategies to leverage the advantages of exponentiation in order to produce accurate and efficient calculations with exponents in Python.
Choosing the Right Method
Using the **
Operator for Simplicity:
- The
**
operator is concise and easy to use for general-purpose exponentiation. - It is suitable for most basic calculations involving integers and floats.
base = 3
exponent = 4
result = base ** exponent
print(result) # Output: 81
Use pow()
for Compatibility and Modulo Operations:
- The built-in pow() function is useful when you need compatibility with other built-in functions or when performing modular exponentiation.
- Modular exponentiation (i.e., (a ** b) % c) can be performed efficiently using the third argument of pow().
base = 5
exponent = 3
mod = 13
result = pow(base, exponent, mod)
print(result) # Output: 8
Use math.pow() for Floating-Point Exponentiation:
- It is specifically designed for floating-point calculations and always returns a float.
- This function is part of the math module, which includes many other mathematical functions that can be useful in scientific and engineering applications.
import math
base = 2.0
exponent = 3.0
result = math.pow(base, exponent)
print(result) # Output: 8.0
Performance Considerations
Use numpy
for Large-Scale Numerical Computations:
- The numpy library provides optimized functions for numerical operations, including exponentiation.
- It is highly efficient for operations on large arrays and matrices, making it ideal for scientific computing and data analysis.
import numpy as np
large_base = np.array([2])
large_exponent = 1000
result = np.power(large_base, large_exponent)
print(result) # Output: [10715086071862673209484250490600018105614048117055336074437503883703510511249361224931983788156958581275946729175531468251871452856923140435984577574698574803934567774824230985421074605062371141877954182153046474983581941267398767559165543946077062914571196477686542167660429831652624386837205668069376]
Precompute Exponents When Possible:
- If you need to perform the same exponentiation multiple times, consider precomputing the result and storing it in a variable.
- This can save computation time and improve performance, especially in loops or recursive functions.
base = 2
exponent = 10
precomputed_result = base ** exponent
for _ in range(1000):
result = precomputed_result
# Perform other operations using the result
Memory Usage and Efficiency
Avoid Unnecessary Floating-Point Conversions:
- Use integer exponentiation when possible to avoid the overhead and potential precision issues associated with floating-point arithmetic.
- Reserve math.pow() and similar functions for cases where floating-point precision is required.
# Integer exponentiation
base = 3
exponent = 10
result = base ** exponent # More efficient than using math.pow()
Handle Large Numbers Carefully:
- Python’s int type can handle arbitrarily large numbers, but operations on very large numbers can be slow and memory-intensive.
- For extremely large numbers, consider using specialized libraries such as gmpy2 for faster performance.
import gmpy2
base = gmpy2.mpz(2)
exponent = 1000
result = gmpy2.powmod(base, exponent, gmpy2.mpz(10**10))
print(result)
Best Practices for Writing Efficient Code
Writing efficient code for exponentiation in Python involves choosing the right method (**
operator, pow(), math.pow()), optimizing for performance using libraries like numpy, handling edge cases carefully (floating-point precision, overflow errors), and using functions and modules for better organization and reusability.
Use Functions and Modules
- Encapsulate repeated exponentiation logic in functions to improve code readability and maintainability.
- Use Python’s standard libraries and third-party modules for well-tested and optimized implementations.
def calculate_exponent(base, exponent):
return base ** exponent
result = calculate_exponent(3, 4)
print(result) # Output: 81
Profile and Optimize
- Employ profiling utilities like as timeit and cProfile to locate performance bottlenecks in your code.
- Only those sections of your code that significantly affect performance should be optimized.
import timeit
setup = "base = 2; exponent = 1000"
statement = "result = base ** exponent"
time_taken = timeit.timeit(stmt=statement, setup=setup, number=100000)
print(f"Time taken: {time_taken} seconds")
Common Pitfalls and How to Avoid Them
Understanding common pitfalls in Python when working with exponents is crucial for writing robust and efficient code, as they can lead to incorrect results or performance issues.
Dealing with Floating-Point Precision
Floating-point numbers are represented in a way that can lead to precision issues, especially when dealing with very large or very small numbers. This can cause inaccuracies in calculations. For instance:
result = 10 ** 20
print(result) # Output: 100000000000000000000
result = math.pow(10, 20)
print(result) # Output: 1e+20
To avoid these issues, you can use the decimal module for arbitrary precision arithmetic when high precision is required:
from decimal import Decimal, getcontext
getcontext().prec = 50 # Set precision to 50 decimal places
base = Decimal(2)
exponent = Decimal(1000)
result = base ** exponent
print(result)
Avoiding Overflow Errors
Exponentiation can result in extremely large numbers, leading to overflow errors in fixed-precision systems or performance and memory issues even in arbitrary-precision systems like Python’s int. Here’s an example:
try:
result = 2 ** 10000
except OverflowError as e:
print(f"OverflowError: {e}")
To handle large numbers effectively, use appropriate data types and algorithms, and consider using modular arithmetic to keep numbers manageable:
import gmpy2
base = gmpy2.mpz(2)
exponent = 1000
modulus = gmpy2.mpz(10**10)
result = gmpy2.powmod(base, exponent, modulus)
print(result)
Handling Negative and Fractional Exponents
Negative and fractional exponents can lead to unexpected results or errors, especially when used with integer bases. For example:
base = 16
negative_exponent = -2
fractional_exponent = 0.5
result_negative = base ** negative_exponent
result_fractional = base ** fractional_exponent
print(result_negative) # Output: 0.00390625
print(result_fractional) # Output: 4.0
Ensure that the base and exponent types are appropriate for the operation and use the math module functions when dealing with non-integer exponents:
import math
base = 16
fractional_exponent = 0.5
result = math.pow(base, fractional_exponent)
print(result) # Output: 4.0
Performance Issues with Large-Scale Exponentiation
Exponentiation with large bases and exponents can be computationally expensive and memory-intensive, leading to performance bottlenecks. For instance:
base = 2
exponent = 100000
result = base ** exponent # This can be slow and memory-intensive
Use optimized libraries like numpy
for large-scale numerical computations:
import numpy as np
large_base = np.array([2])
large_exponent = 1000
result = np.power(large_base, large_exponent)
print(result)
Incorrect Use of Functions
Using the wrong function for a specific type of exponentiation can lead to incorrect results or reduced performance. For example:
result = 2 ** 3 ** 2 # This is interpreted as 2 ** (3 ** 2)
print(result) # Output: 512
Use parentheses to explicitly define the order of operations:
result = (2 ** 3) ** 2
print(result) # Output: 64
Conclusion
Python’s developers have strategically built robust exponentiation capabilities into the language, providing users with a versatile toolkit for mathematical computations, featuring a range of approaches, from simple ** operator usage to advanced negative exponents and complex number operations. Developers may fully utilize exponentiation in Python to enable precise and efficient solutions in a variety of scientific, financial, and computational domains by following optimization methodologies, utilizing relevant modules, and avoiding frequent mistakes.
Don’t miss this chance to develop your abilities and further your career. Come learn Python with us! We offer an extensive and captivating free course that is suitable for all levels.
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/06/a-comprehensive-guide-on-exponents-in-python/