Introduction
Welcome to “A Comprehensive Guide to Python Docstrings,” where we embark on a journey into documenting Python code effectively. Docstrings are pivotal in enhancing code readability, maintainability, and collaboration among developers. In this detailed exploration, we will unravel the intricacies of Python docstrings, covering their importance, types, and how to write python docstrings. Whether you’re a beginner seeking to understand the basics or an experienced developer aiming to refine your documentation skills, this guide is your go-to resource for mastering the art of Python docstrings.
Table of contents
What is Python Docstrings?

Python Docstrings are pivotal in code documentation, elevating code readability and comprehension. Nestled within code, these triple-quoted strings act as a window into the intricacies of modules, functions, classes, and methods. A Python Docstring, enclosed in triple quotes, is the initial statement in a module, function, class, or method. It is a documentation tool highlighting the code’s purpose and functionality.
Importance of Python Docstrings
Python docstrings are crucial for various reasons:
- Documentation: Docstrings function as code documentation, articulating the purpose, usage, and behavior of functions, classes, modules, or methods. This documentation serves as a guide for code users and maintainers.
- Readability: Well-crafted docstrings enhance code readability, offering a concise understanding of code functionality. This becomes paramount in collaborative projects where multiple developers work on the same codebase.
- Auto-generated documentation: Docstrings aid documentation generation tools like Sphinx, automating documentation creation in formats like HTML or PDF. This streamlines the process of maintaining up-to-date project documentation.
- IDE support: Integrated Development Environments (IDEs) leverage docstrings to provide contextual assistance and suggestions during code writing. This includes function signatures, parameter information, and brief descriptions, facilitating correct code usage.
- Testing and debugging: Docstrings furnish information about expected inputs and outputs, aiding in testing and debugging. Developers can rely on this information to write effective test cases and understand the expected behavior of functions or methods.
- API documentation: For libraries intended for external use, docstrings serve as API documentation. They detail how to interact with the code, expected inputs and outputs, and other pertinent information for users.
Embark on your coding adventure now! Join our complimentary Python course and effortlessly enhance your programming prowess by mastering essential sorting techniques. Start today for a journey of skill development!
Types of Docstrings
- Single-line Docstrings : Single-line docstrings, concise and suitable for brief documentation, are commonly used for simple functions or modules.
- Multi-line Docstrings: Multi-line docstrings, offering detailed documentation, are recommended for complex functions, classes, or modules, providing a comprehensive overview.
How to Write Python Docstrings?
Triple Quotes: Use triple double-quotes (“””) for docstrings to allow multiline docstrings.
def example_function(parameter):
"""
This is a docstring for the example_function.
Parameters:
- parameter: Describe the purpose and expected type of the parameter.
Returns:
Describe the return value and its type.
Raises:
Document any exceptions that can be raised and under what conditions.
"""
# Function implementation here
Writing Single-line Docstrings: Craft single-line docstrings by summarizing the code entity’s purpose in one line. This format suits straightforward functions or modules.
def add_numbers(a, b):
"""Add two numbers."""
return a + b
Sections in Docstrings
Organize docstrings into sections for clarity. Common sections include:
- Parameters: Describe parameters and their types.
- Returns: Explain the return value and its type.
- Raises: Document exceptions the function or method may raise.
- Examples: Provide usage examples if necessary.
def divide_numbers(dividend, divisor):
"""
Divide two numbers.
Parameters:
- dividend (float): The number to be divided.
- divisor (float): The number by which the dividend is divided.
Returns:
float: The result of the division.
Raises:
ValueError: If the divisor is zero.
"""
if divisor == 0:
raise ValueError("Cannot divide by zero.")
return dividend / divisor
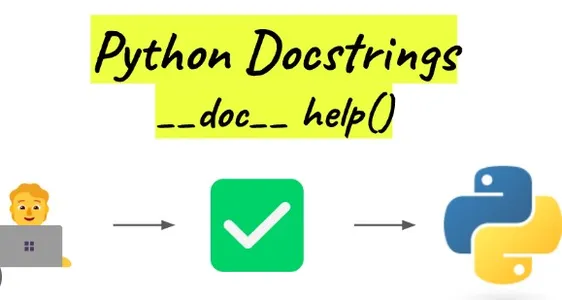
Comments:
- Comments are for adding explanatory notes within the code.
- They begin with the # symbol.
- Ignored by the Python interpreter during runtime, comments are solely for human readers.
# This is a single-line comment
x = 10 # This is an inline comment
Docstrings:
- Docstrings document functions, modules, classes, or methods in a structured way.
- Enclosed in triple-quotes (”’ or “””), they can span multiple lines.
- Accessible at runtime using the .__doc__ attribute.
- Used for automated documentation generation tools.
def example_function(arg1, arg2):
"""
This is a docstring for example_function.
Args:
arg1: The first argument.
arg2: The second argument.
Returns:
The result of the operation.
"""
return arg1 + arg2
Accessing Docstrings
- Using the __doc__ Attribute: Access docstrings within the code using the __doc__ attribute, holding the docstring of the associated code entity.
- Using the help() Function: The help() function provides interactive help and can access docstrings by passing the code entity as an argument.
- Using the pydoc Module: The pydoc module generates documentation based on docstrings present in the code.
Docstring Formats
- reStructuredText: A lightweight markup language for readable and structured docstrings, commonly used for Python documentation.
- Google Style: Google-style docstrings follow a specific format with sections like Args, Returns, and Examples, widely adopted in the Python community.
- Numpydoc: Numpydoc, commonly used in the scientific Python community, extends reStructuredText with conventions for documenting NumPy-related code.
- Epytext: Epytext is a markup language supporting Python module, class, and function documentation.
- Doctest Module: The doctest module allows the inclusion of testable examples within docstrings, ensuring documentation accuracy.
- Pydoc: Pydoc is a documentation generation tool extracting information from docstrings to create HTML documentation.
- Sphinx: Sphinx, a powerful documentation generation tool, supports various output formats, enabling professional-looking documentation.
- Pylint: Pylint, a static code analysis tool, checks for adherence to coding standards, including the presence and quality of docstrings.
Conclusion
Mastering Python docstrings is imperative for effective code documentation. This journey transforms your coding practices from basics to choosing the right format and utilizing tools.
Proper docstring usage significantly contributes to code maintainability, collaboration, and project success. Investing time in crafting meaningful docstrings is an investment in the long-term viability of your codebase.
Embark on an exhilarating coding journey today! Unleash the power of programming by enrolling in our complimentary Python course. Master essential sorting techniques effortlessly and watch your coding skills soar to new heights. Don’t miss this opportunity to supercharge your programming journey – enroll now and let the coding magic begin!
Frequently Asked Questions
A. A Python Docstring is a string literal, enclosed in triple quotes, serving as the first statement in a module, function, class, or method. It acts as documentation, providing insights into the purpose and functionality of the code.
A. Python Docstrings are crucial for documentation, enhancing code readability, and serving as a guide for users and maintainers. They contribute to auto-generated documentation, IDE support, testing, debugging, and API documentation.
A. Python Docstrings use triple double-quotes (“””) for multiline docstrings. Writing involves summarizing the purpose, describing parameters, returns, and raising exceptions, organized into sections.
A. Python Docstrings can be accessed using the __doc__ attribute of the associated code entity. The help() function and the pydoc module are also tools for accessing docstrings.
Related
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/01/python-docstrings/