Introduction
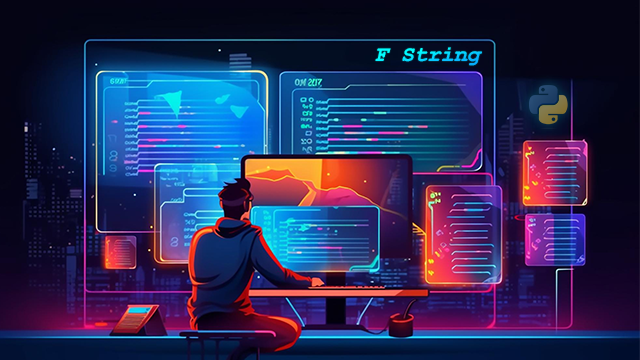
String formatting is an essential aspect of programming, allowing us to manipulate and present data in a readable and organized manner. In Python, several methods are available for string formatting, each with its advantages and use cases. One such method is f-strings, which provide a concise and efficient way to format strings. In this article, we will explore the power of f-strings and learn how to use them effectively in Python.
Table of contents
What are f-strings?
F-strings, also known as formatted string literals, were introduced in Python 3.6. They provide a concise and readable way to embed expressions inside string literals, making string formatting more intuitive and efficient. The ‘f’ prefix denotes f-strings before the opening quotation mark of a string.
Benefits of using f-strings in Python
There are several benefits to using f-strings in Python:
- Readability: F-strings make the code more readable by allowing us to embed expressions directly within the string, eliminating the need for complex concatenation or formatting operations.
- Conciseness: F-strings provide a concise syntax for string formatting, reducing the amount of code required compared to other methods.
- Performance: F-strings are faster than string formatting methods like %-formatting or str.format(). They are evaluated at runtime and can be optimized by the Python interpreter.
- Flexibility: F-strings support various formatting options, allowing us to customize the output according to our requirements.
Basic Syntax of f-strings
To create an f-string, prefix the string with the letter ‘f’. You can embed expressions inside the string by enclosing them in curly braces {}. The expressions will be evaluated and replaced with their values at runtime.
Code:
name = "Alice"
age = 25
print(f"My name is {name} and I am {age} years old.")
Output:
My name is Alice and I am 25 years old.
In the above example, the expressions `{name}` and `{age}` are evaluated and replaced with their respective values when the string is printed.
String interpolation with f-strings
F-strings allows us to interpolate variables directly into the string. This makes the code more readable and eliminates the need for explicit type conversions.
Code:
name = "Bob"
age = 30
print(f"Hello, {name}! You are {age} years old.")
Output:
Hello, Bob! You are 30 years old.
Formatting Expressions With f-strings
F-strings support various formatting options to customize the output. Let’s explore some of these options:
Specifying field width and alignment
We can specify the field width and alignment of the interpolated values using the colon ‘:’ followed by the format specifier.
Code:
name = "Charlie"
age = 35
print(f"Name: {name:<10} Age: {age:>5}")
Output:
Name: Charlie Age: 35
In the above example, the field width for the name is set to 10 characters and left-aligned, while the field width for the age is set to 5 characters and right-aligned.
Precision and rounding with f-strings
We can control the precision and rounding of floating-point numbers using the format specifier.
Code:
pi = 3.141592653589793
print(f"Value of pi: {pi:.2f}")
Output:
Value of pi: 3.14
In the above example, the precision of the floating-point number is set to 2 decimal places.
Handling numeric values with f-strings
F-strings provide various options for formatting numeric values, such as adding commas for thousands of separators or displaying numbers in different number systems.
Code:
number = 1000000
print(f"Formatted number: {number:,}")
Output:
Formatted number: 1,000,000
In the above example, the comma format specifier adds thousands of separators to the number.
Working with dates and times using f-strings
F-strings can also format dates and times using the datetime module.
Code:
from datetime import datetime
now = datetime.now()
print(f"Current date and time: {now:%Y-%m-%d %H:%M:%S}")
Output:
Current date and time: 2022-01-01 12:34:56
In the above example, the format specifier displays the current date and time in the specified format.
Advanced Techniques with F-strings
In addition to the basic usage, f-strings offer advanced techniques that enhance their functionality and flexibility.
Using f-strings with dictionaries and objects
F-strings can be used to interpolate values from dictionaries and objects by accessing their keys or attributes.
Code:
person = {"name": "Alice", "age": 25}
print(f"Name: {person['name']} Age: {person['age']}")
Output:
Name: Alice Age: 25
In the above example, the values from the dictionary are interpolated into the string using f-strings.
Conditional statements and loops in f-strings
F-strings support conditional statements and loops, allowing us to perform dynamic string formatting based on certain conditions.
Code:
name = "Bob"
age = 30
is_adult = True
print(f"{name} is {'an adult' if is_adult else 'a minor'} of age {age}.")
Output:
Bob is an adult of age 30.
In the above example, the f-string uses a conditional statement to determine whether the person is an adult or a minor based on the value of `is_adult`.
Nesting f-strings within f-strings
F-strings can be nested within each other to create more complex string formatting.
Code:
name = "Charlie"
age = 35
print(f"My name is {name} and I am {age} years old. {'Wow, that's young!' if age < 40 else 'Wow, that's old!'}")
Output:
My name is Charlie and I am 35 years old. Wow, that’s young!
In the above example, the f-string is nested within another f-string to add a comment based on the age conditionally.
Escaping characters in f-strings
To include curly braces {} in an f-string without interpolation, we can escape them by doubling them.
Code:
name = "Alice"
print(f"Her name is {name} and she said {{Hello}}.")
Output:
Her name is Alice and she said {Hello}.
In the above example, the double curly braces {{}} are used to escape the inner curly braces and treat them as literal characters.
Comparison to Other String Formatting Methods
Comparison to %-formatting
%-formatting is an older method of string formatting in Python. While supported, f-strings offer a more concise and readable syntax.
Code:
name = "Alice"
age = 25
print("My name is %s and I am %d years old." % (name, age))
Output:
My name is Alice and I am 25 years old.
In the above example, the %-formatting syntax requires explicit type conversions and is less readable than f-strings.
Comparison to str.format()
The str.format() method is another method of string formatting in Python. While it offers more flexibility than %-formatting, f-strings provide a more concise and intuitive syntax.
Code:
name = "Alice"
age = 25
print("My name is {} and I am {} years old.".format(name, age))
Output:
My name is Alice and I am 25 years old.
In the above example, the str.format() method requires placeholders {} and positional arguments, making the code less readable compared to f-strings.
Comparison to Template strings
Template strings provide a simple and safe way to perform string substitution. However, they lack the flexibility and advanced features offered by f-strings.
Code:
from string import Template
name = "Alice"
age = 25
template = Template("My name is $name and I am $age years old.")
print(template.substitute(name=name, age=age))
Output:
My name is Alice and I am 25 years old.
In the above example, the Template string requires explicit substitution using the `substitute()` method, making it less concise than f-strings.
Conclusion
F-strings are a powerful and efficient way to format strings in Python. They provide a concise and readable syntax, making string formatting more intuitive and enjoyable. By mastering f-strings, you can enhance your Python code and improve the readability and maintainability of your projects. So go ahead, start using f-strings in your Python code, and experience the benefits they offer in string formatting.
Related
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/01/mastering-f-strings-in-python/