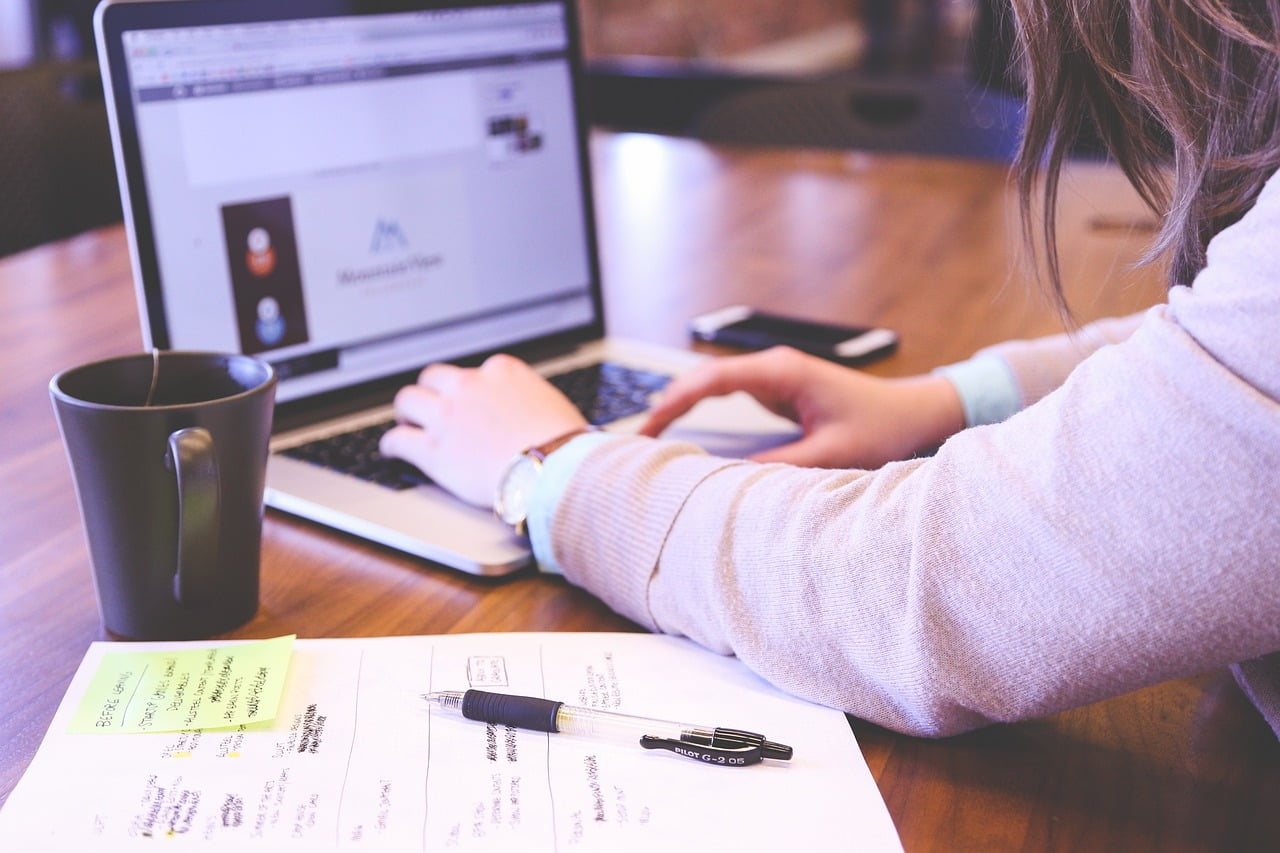
Demand for data scientists is growing. The BLS reports that the number of data science jobs will grow 35% between 2022 and 2032, which is much faster than the average of all professions. As the need for data scientists grows, so will the demand for technologies they rely on.
Are you considering a career in data science? There are a number of things that you are going to need to be aware of. One of the most important things to consider is the importance of knowing the right programming language.
We have talked about some of the most popular programming languages for data scientists. As we mentioned, Python tends to be the most popular.
However, there are a number of other great articles for data scientists to try as well. C++ is a great one to look at. One survey found that 22.42% of all programmers know how to use C++.
Students will learn the fundamentals of computer programming. They will write programs that process text and generate output based on input data.
Class assignments are designed to help you become comfortable designing and building software in C++. They will play with control structures, string processing and recursion.
Homework assignments will be submitted online here ref.page . The instructor will not accept work that has been written or printed on paper.
Functions
Functions are a vital part of any programming language. They allow programmers to reuse code, making the code easier to read and understand. Functions are also helpful in creating complex programs and automating tasks.
Functions in C++ are composed of two main elements: declarations and definitions. A function declaration informs the compiler what the function looks like without defining its implementation. The actual code that runs when the function is called is defined in a separate block of code, known as a function definition.
A function can take a list of values, known as parameters, into which it can operate. When an argument is passed into a function, the corresponding value is stored in a variable with a reference to that parameter. The variable is then accessed and modified within the function. When the function is finished, the corresponding value is returned to the calling program.
Variables
Variables in C++ store data in memory locations, which can be modified during program execution. They can be of different types such as int, float, boolean, and char. They can also have different scopes such as global and local.
A variable must have a name, and this is its identification in the code. It must consist of alphabets (both uppercase and lowercase) and digits, and cannot start with a underscore (_). Its type must be specified when it is declared.
Usually, a variable is defined and declared in the same line of the code. Its initialization is also a part of its declaration. However, it can be declared any number of times but must be initialized once; otherwise, the compiler would waste memory. This is called variable initialization. It is important to understand how variables work to write efficient code. This knowledge will help you to create more powerful programs and efficiently use memory resources.
Objects
In C++, objects are the blueprints for creating real-world entities such as trees, chairs, dogs, and cars. They contain data variables and member functions. They can also have private and public data members and constructors. Private data members cannot be accessed directly from outside the class, but can be accessed through public member functions.
Objects allow you to model real-world entities within your programs, which makes them a core feature of the language. The use of objects and classes can make your code more efficient and easier to read. But the concept can be tricky to grasp for beginners and even experienced programmers.
C++ is a powerful programming language that allows you to create efficient and well-organized code. It also supports the use of objects and templates, which allow you to construct complex expressions. But these features can be confusing for new programmers, especially when trying to understand how objects and classes work.
Arrays
Arrays are a way of storing multiple values in a single variable. They can be used to store a collection of data items of the same type (int, char, float, etc). This allows us to save space in memory and makes the code easier to read.
Unlike variables, which are assigned at run time, an array is allocated at compile time and the size of the array is fixed. An array can have one or more dimensions. One-dimensional arrays are often referred to as vectors, while two dimensional arrays are called matrices.
You access an array element by referring to the index number inside square brackets []. Counting in computer science starts at zero, so the first item in an array has index 0, and so on. Similarly, in functions, you can pass an array by its name or by its address, which is called passing by reference.
Strings
Strings are a sequence of characters that can be used to store text. They can be manipulated in many ways. For example, they can be concatenated with other strings using the strcat() function. They can also be compared using the string compare() function. In addition to these functions, string classes provide more advanced features than character arrays. Strings can be indexed like arrays and are usually stored in the object memory. They can be accessed by using the cin keyword or by calling the string class function getline().
The string class provides a method called c_str() that returns a pointer to a char constant equivalent to the contents of the C string. This can be useful if you want to pass a string object to a function that accepts only the C-style string. However, you should keep in mind that a C-style string pointer cannot tell you whether the data it points to has valid values or not.
Object-Oriented Programming
C++ is an object-oriented programming language, which means that it uses objects and classes to represent real-world entities. This approach allows for code reusability, helps to solve complex problems, and makes it easier to maintain the code. It also provides flexibility for a growing application with the concepts of encapsulation, inheritance, and polymorphism.
A class is a blueprint, while an object is a specific instance of that class. A class can have static members, which are shared by all the objects of that class, or methods, which are specific to each instance of the class.
Inheritance allows for passing traits from parent to child. For example, a SportsCar can inherit the ability to start and stop from its Car parent. This is known as abstraction, and it means that you don’t need to know how the car works inside; you just need to press the “start” button.
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.smartdatacollective.com/fundamentals-of-c-programming-for-data-scientists/