Welcome to the Python Error Handling MCQ Quiz! Error handling is an essential aspect of programming, and Python provides powerful mechanisms to manage exceptions and errors that may occur during program execution. This quiz aims to test your understanding of various concepts related to error handling in Python, including try
and except
blocks, raising and catching exceptions, using finally
blocks, and more. Each question is multiple-choice, with only one correct answer. Take your time to carefully read each question and choose the best option. Let’s begin and explore Python error handling together!
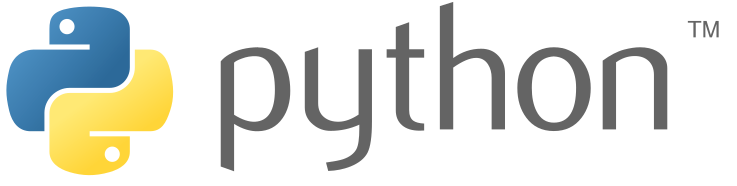
Q1. What is the purpose of the try block in Python error handling?
a) To define the block of code where an exception may occur
b) To catch and handle exceptions that occur within the block
c) To ensure that the code executes without any errors
d) To terminate the program if an exception occurs
Answer: a
Explanation: The try block defines a block of code in which exceptions may occur.
Q2. Which keyword is used to catch exceptions in Python?
a) try
b) catch
c) except
d) handle
Answer: c
Explanation: The except keyword is used to catch and handle exceptions in Python.
Q3. What is raised when a Python program encounters an error during execution?
a) Error
b) Exception
c) Fault
d) Bug
Answer: b
Explanation: In Python, errors during execution are represented as exceptions.
Q4. Which of the following is NOT a standard Python exception?
a) KeyError
b) ValueException
c) IndexError
d) TypeError
Answer: b
Explanation: ValueException is not a standard Python exception. It should be ValueError.
Q5. How can you handle multiple exceptions in a single except block?
a) Separate the exceptions using commas
b) Use nested except blocks
c) Use the except keyword only once
d) It is not possible to handle multiple exceptions in a single except block
Answer: a
Explanation: You can separate multiple exceptions using commas in a single except block.
Q6. Which Python keyword is used to raise an exception manually?
a) throw
b) raise
c) exception
d) trigger
Answer: b
Explanation: The raise keyword is used to raise exceptions manually in Python.
Q7. What does the finally block in Python error handling ensure?
a) It ensures the code within it will always execute, regardless of whether an exception occurs or not.
b) It ensures the program will terminate if an exception occurs.
c) It ensures that the program will skip executing the code if an exception occurs.
d) It ensures that only the code within the finally block will execute if an exception occurs.
Answer: a
Explanation: The finally block ensures that the code within it will always execute, regardless of whether an exception occurs or not.
Q8. Which of the following is true about the else block in Python error handling?
a) It is executed if an exception occurs.
b) It is executed if no exception occurs in the try block.
c) It handles exceptions occurring in the try block.
d) It is executed instead of the finally block.
Answer: b
Explanation: Python executes the else block if no exception occurs in the try block.
Q9. What is the purpose of the assert statement in Python?
a) To handle exceptions
b) To terminate the program
c) To check if a condition is true
d) To raise an exception
Answer: c
Explanation: The assert statement checks if a condition is true in Python. If the condition is false, it raises an AssertionError.
Q10.Which of the following methods is NOT a commonly used method of the Exception class in Python?
a) __str__()
b) __init__()
c) __cause__()
d) __repr__()
Answer: c
Explanation: __cause__() is not a method of the Exception class. It is used to get the cause of the exception.
Q11. Which of the following statements is true about the except clause in Python?
a) It is mandatory in a try-except block.
b) It catches all exceptions by default.
c) It must be placed before the try block.
d) It can specify the type of exceptions to catch.
Answer: d
Explanation: The except clause can specify the type of exceptions to catch.
Q12. What is the output of the following code?
try:
x = 10 / 0
except ZeroDivisionError:
print("Division by zero")
finally:
print("Finally block")
a) Division by zero
Finally block
b) Finally block
c) Division by zero
d) ZeroDivisionError
Answer: a
Explanation: The code will raise a ZeroDivisionError, catch it, print “Division by zero”, and then execute the finally block printing “Finally block”.
Q13. Which of the following keywords is used to handle the exception block in Python?
a) hand
b) rescue
c) except
d) catch
Answer: c
Explanation: except is used to handle exception blocks in Python.
Q14. What does the following Python code do?
try:
# Some code that may raise an exception
except:
pass
a) It raises an exception.
b) It catches all exceptions and ignores them.
c) It terminates the program.
d) It handles exceptions gracefully.
Answer: b
Explanation: This code catches all exceptions and ignores them due to the pass statement.
Q15. What happens if an exception occurs in the finally block itself?
a) The exception is caught by the except block.
b) The program terminates.
c) The exception propagates up the call stack.
d) The exception is ignored.
Answer: c
Explanation: If an exception occurs in the finally block itself, it propagates up the call stack.
Q16. Which of the following is NOT a common built-in exception in Python?
a) KeyError
b) FileNotFoundError
c) IndexError
d) SyntaxError
Answer: d
Explanation: SyntaxError is a common syntax error, but it’s not a built-in exception class.
Q17. What is the purpose of the sys.exc_info() function in Python?
a) It raises an exception.
b) It returns information about the current exception being handled.
c) It terminates the program.
d) It prints the traceback of the exception.
Answer: b
Explanation: sys.exc_info() returns a tuple of information about the current exception being handled in Python.
Q18. Which keyword is used to re-raise the last exception that was caught in Python?
a) rethrow
b) rethrow_last
c) raise_last
d) raise
Answer: d
Explanation: In Python, developers use the raise keyword to re-raise the last exception that was caught.
Q19. What is the output of the following code?
try:
raise Exception("An error occurred")
except Exception as e:
print(e)
finally:
print("Finally block")
a) An error occurred
Finally block
b) Finally block
c) An error occurred
d) Exception
Answer: a
Explanation: The code raises an Exception, catches it, prints the error message, and then executes the finally block.
Q20. Which statement is true about handling exceptions in Python?
a) An exception handler can catch exceptions raised by functions it calls.
b) An exception handler cannot catch exceptions raised by functions it calls.
c) An exception handler only catches exceptions raised in the same block.
d) An exception handler can only catch exceptions of the same type.
Answer: a
Explanation: An exception handler can catch exceptions raised by functions it calls.
Q21. What is the purpose of the traceback module in Python?
a) It allows you to customize error messages.
b) It prints detailed information about exceptions.
c) It handles exceptions in multi-threaded applications.
d) It raises exceptions based on user-defined conditions.
Answer: b
Explanation: The traceback module provides functions to print detailed information about exceptions.
Q22. Which of the following statements is true about the assert statement in Python?
a) It is used to handle exceptions.
b) It terminates the program if a condition is true.
c) It raises an exception if a condition is false.
d) It is similar to the try-except block.
Answer: c
Explanation: The assert statement raises an AssertionError if a condition is false.
Q23. How can you create a custom exception class in Python?
a) By inheriting from the Exception class
b) By using the throw keyword
c) By using the custom_exception keyword
d) By defining a function with the name of the exception
Answer: a
Explanation: You can create a custom exception class by inheriting from the Exception class.
Q24. What does the sys.exit() function do in Python?
a) Raises an exception
b) Terminates the program
c) Prints a message to the console
d) Handles exceptions
Answer: b
Explanation: sys.exit() terminates the program.
Q25. Which of the following is true about the else block in Python error handling?
a) It is executed if an exception occurs.
b) It handles exceptions occurring in the try block.
c) It is executed only if an exception occurs.
d) It is executed if no exception occurs in the try block.
Answer: d
Explanation: If no exception occurs in the try block, Python executes the else block.
Q26. What is the purpose of the raise statement in Python?
a) To catch exceptions
b) To ignore exceptions
c) To re-raise exceptions
d) To terminate the program
Answer: c
Explanation: In Python, developers use the raise statement to re-raise exceptions.
Q27. Which of the following is NOT a standard Python exception?
a) SyntaxError
b) ZeroDivisionError
c) OverflowError
d) RuntimeError
Answer: c
Explanation: OverflowError is a standard Python exception, but it’s not as common as the others listed.
Q28. What is the purpose of the try-except-else block in Python?
a) To catch exceptions and execute alternative code
b) To execute code that should always run
c) To handle exceptions and execute code if no exception occurs
d) To terminate the program if an exception occurs
Answer: c
Explanation: In Python, developers use the try-except-else block to handle exceptions and execute code if no exception occurs.
Q29. Which keyword is used to define a custom exception class in Python?
a) catch
b) raise
c) class
d) exception
Answer: c
Explanation: In Python, developers use the class keyword to define custom exception classes.
Q30. What is the purpose of the finally block in Python error handling?
a) To handle exceptions
b) To raise exceptions
c) To ensure that certain code will always be executed
d) To terminate the program
Answer: c
Explanation: The finally block ensures that certain code executes, regardless of whether an exception occurs.
Q31. Which of the following exceptions is raised when trying to access an index in a list that does not exist?
a) ValueError
b) KeyError
c) IndexError
d) TypeError
Answer: c
Explanation: Attempting to access an index that is out of range in a sequence like a list raises an IndexError.
Q32. In a try-except block, can we have multiple except blocks?
a) No, only one except block is allowed
b) Yes, but only if they handle different types of exceptions
c) Yes, regardless of the types of exceptions they handle
d) Yes, but only if they are nested within each other
Answer: b
Explanation: In Python, ordering multiple except blocks from most specific to least specific is considered good practice because Python checks them in order and executes the first matching block.
Q33. Which of the following statements is true regarding the order of except blocks in a try-except construct?
a) The order of except blocks doesn’t matter
b) The most specific exception handlers should come first
c) The most general exception handlers should come first
d) Python raises a SyntaxError if except blocks are not in the correct order
Answer: b
Explanation: In Python, it’s good practice to order multiple except blocks from most specific to least specific because Python checks them in order and executes the first matching block.
Congratulations on completing the Python Error Handling MCQ Quiz! We hope this quiz has helped reinforce your understanding of Python error handling concepts and techniques. Managing exceptions effectively is crucial for writing robust and reliable Python code. By mastering error handling, you can ensure that your programs gracefully handle unexpected situations and provide meaningful feedback to users. Keep practicing and exploring Python’s error handling mechanisms to become a proficient Python developer. If you have any questions or want to delve deeper into any topic, don’t hesitate to continue your learning journey. Happy coding!
You can also enroll in out free Python Course Today!
Read our more articles related to MCQs in Python:
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/02/30-mcqs-on-python-error-handling-try-except/