Introduction
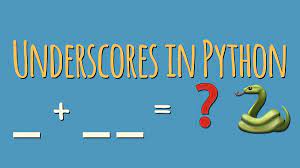
Python, a versatile and dynamic programming language, relies on symbols to convey meaning and functionality. This exploration delves into the significance of one such symbol: the underscore (_). In Python, the underscore (_) is a versatile symbol. It is commonly used as a throwaway variable, a placeholder in loops, and for internal naming conventions. In the interactive interpreter, a single underscore represents the result of the last expression evaluated. Additionally, in internationalization, it serves as an alias for the gettext() function for marking strings for translation. The underscore’s adaptability makes it a handy tool in various coding scenarios.
Table of contents
The Single Underscore
The single underscore serves multifaceted roles within Python’s syntax. It acts as a placeholder, emphasizing the importance of the variable or operation it represents. Here are some common use cases:
Underscore As a Variable
1. Temporary or Unimportant Variable
Using as a placeholder for a temporary or unimportant variable. For example:
_, result = some_function_returning_tuple()
2. “I don’t care” or “Throwaway” Variable
Use this when you don’t care about the value of a particular variable. For example, you can use it in unpacking sequences to ignore elements you don’t need.
_, important_value = get_data()
3. Private Variables
It is sometimes used as a convention to indicate that a variable is intended for internal use only (similar to a “private” variable).
_internal_variable = 42
Underscore In Interpreter
In the Python interpreter, a single underscore (`_`) has a special role as a placeholder for the result of the last expression when used in an interactive session. It is primarily used in the context of the interactive Python shell (REPL – Read-Eval-Print Loop).
Here are a couple of common use cases:
1. Storing the result of the last expression
>>> x = 5
>>> x + 3
8
>>> _
8
In this example, the underscore `_` is used to reference the result of the last expression (`x + 3`), which is 8.
2. Ignoring a value
>>> _, y, _ = (1, 2, 3)
>>> y
2
Here, the underscores are used as a convention to indicate that the unpacked values are not interesting, and only the middle value (`y`) is kept.
Underscore In Looping
A single underscore can be used as a conventional way to indicate intentional unused variables when you’re not utilizing the loop variable within a loop.
for _ in range(5):
# do something without using the loop variable
Underscore for Internationalization and Translation
It is sometimes used as a name for a variable when the variable is just a placeholder, and its value will not be used. This is common in internationalization and translation functions, where the translator doesn’t need the variable’s value.
_('This is a translatable string')
Underscore for Ignoring Values
Discards unwanted values during unpacking, enhancing code clarity.
Remember that using a single underscore for variable names is mostly a convention, and Python won’t treat it differently from other variable names. It’s a way for programmers to communicate to others (and to themselves) about the intended use of a variable.
The Double Underscore
The double underscore, or dunder, plays a pivotal role in Python’s naming conventions and encapsulation mechanisms.
Name Mangling
In a class definition, when you prefix a name with double underscores (e.g., __variable
), Python utilizes name mangling to render the name more unique, preventing inadvertent name conflicts in subclasses. Programmers often employ this technique to pseudo-privatize attributes or methods within a class, despite Python lacking true private members.
class MyClass:
def __init__(self):
self.__private_variable = 42
# Accessing the private variable outside the class
obj = MyClass()
print(obj.__private_variable) # This would raise an AttributeError
Special methods (dunder methods)
In Python, programmers commonly use double underscores as a convention for special methods, referred to as “dunder” methods (short for double underscores). These methods carry specific meanings within the language, serving purposes like operator overloading or defining particular behaviors for objects.
class MyClass:
def __str__(self):
return "This is a custom string representation"
obj = MyClass()
print(obj) # This will call the __str__ method
Magic Constants
Some double underscore names are used as magic constants, like `__file__` and `__name__`, which provide information about the current module or script.
print(__file__) # Name of the current script
print(__name__) # Name of the current module
Ignored special methods
Sometimes, a double underscore may indicate that a method is intended for internal use and should not be considered part of the public API. This is more of a convention and doesn’t have a specific language-level effect.
These are some common use cases for the double underscore in Python. It’s important to note that using double underscores for name mangling and special methods is a convention, and Python doesn’t enforce strict privacy or access control. Programmers are expected to follow conventions for code readability and maintainability.
Conclusion
Understanding the role and usage of underscores in Python is crucial for any programmer. It enhances the readability of the code and helps in writing efficient and clean code. Though a simple symbol, the underscore significantly impacts Python programming.
Related
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/01/role-of-underscore-in-python/