Introduction
String matching in Python can be challenging, but Pregex makes it easy with its simple and efficient pattern-matching capabilities. In this article, we will explore how Pregex can help you find patterns in text effortlessly. We will cover the benefits of using Pregex, a step-by-step guide to getting started, practical examples, tips for efficient string matching, integration with other Python libraries, and best practices to follow. Whether you are a beginner or an experienced programmer, Pregex can simplify your string-matching tasks and enhance your Python projects.
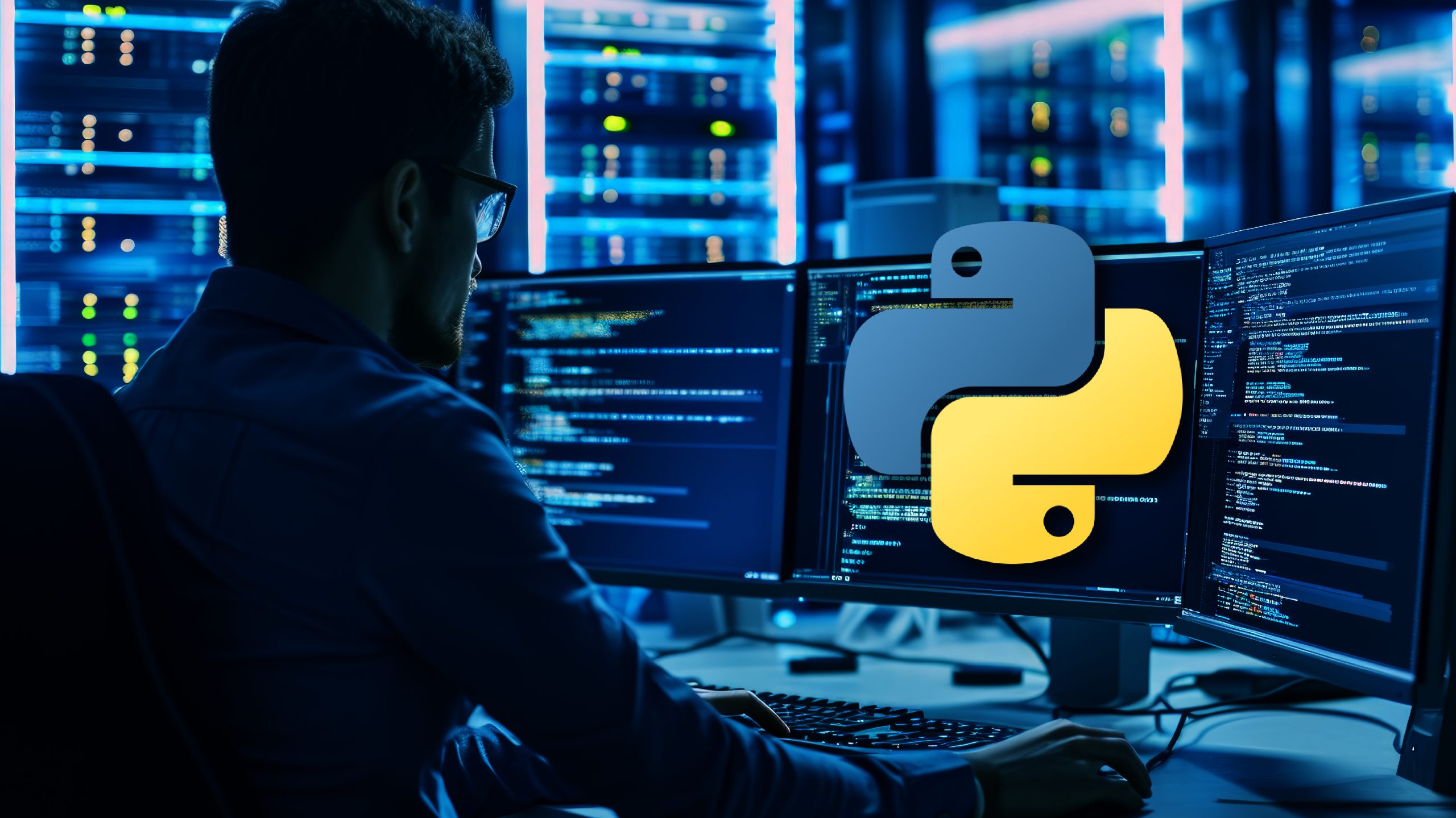
Table of contents
Benefits of Using Pregex for String Matching
Pregex is a Python utility that simplifies the process of identifying patterns in text without requiring knowledge of complex programming. Because it simplifies and manages the code, Pregex benefits novice and seasoned programmers. Pregex makes setting up and applying patterns easy, accelerating development and lowering error rates. Additionally, this accessibility facilitates quicker code updates and debugging, maintaining projects’ flexibility and efficiency.
Getting Started with Pregex in Python
You must first install the library to start using Pregex in your Python project. You can easily install Pregex using pip:
pip install pregex
Basic Pattern Matching
Once you have installed Pregex, you can use it to do basic pattern matching. For example, to check if a string contains a specific word, you can use the following code:
from pregex.core.pre import Pregex
text = "Hello, World!"
pattern = Pregex("Hello")
result = pattern.get_matches(text)
if result:
print("Pattern found!")
else:
print("Pattern not found.")
Output: Pattern found!
Explanation
- Import the Pregex class from the pregex.core.pre module.
- Define the text to search:
- text = “Hello, World!”: This is the text in which we want to find the pattern.
- Create a pattern:
- pattern = Pregex(“Hello”): This creates a Pregex object with the pattern “Hello”.
- Find matches:
- result = pattern.get_matches(text): This uses the get_matches method to find occurrences of the pattern “Hello” in the text.
- Check and print results:
- The if statement checks if any matches were found.
- If matches are found, it prints “Pattern found!”.
- If no matches are found, it prints “Pattern not found.”
Advanced Pattern Matching Techniques
Pregex also supports advanced pattern-matching techniques such as using anchors, quantifiers, grouping, and capturing matches. These techniques allow you to create more complex patterns for matching strings.
Examples of String Matching with Pregex
Matching Email Addresses
text="Hello there, [email protected]"
from pregex.core.classes import AnyButFrom
from pregex.core.quantifiers import OneOrMore, AtLeast
from pregex.core.assertions import MatchAtLineEnd
user = OneOrMore(AnyButFrom("@", ' '))
company = OneOrMore(AnyButFrom("@", ' ', '.'))
domain = MatchAtLineEnd(AtLeast(AnyButFrom("@", ' ', '.'), 3))
pre = (
user +
"@" +
company +
'.' +
domain
)
results = pre.get_matches(text)
print(results)
Output: [‘[email protected]’]
Explanation
- Import necessary Pregex classes:
- AnyButFrom: Matches any character except those specified.
- OneOrMore: Matches one or more occurrences of the preceding element.
- AtLeast: Matches at least a specified number of occurrences of the preceding element.
- MatchAtLineEnd: Asserts that the following pattern must be at the end of the line.
- Define patterns for email parts:
- user: Matches the part before the “@” symbol (OneOrMore(AnyButFrom(“@”, ‘ ‘))).
- company: Matches the part between the “@” symbol and the last dot (OneOrMore(AnyButFrom(“@”, ‘ ‘, ‘.’))).
- domain: Matches the part after the last dot (MatchAtLineEnd(AtLeast(AnyButFrom(“@”, ‘ ‘, ‘.’), 3))).
- Combine the patterns:
- Concatenate user, “@”, company, and domain to form the complete email pattern.
- Find matches in the text:
- Use the get_matches method to find and print any email addresses in the text.
Extracting URLs, Identifying Phone Numbers, and Parsing Data from Text can be done similarly using Pregex.
Also Read: Introduction to Strings in Python For Beginners
Tips for Efficient String Matching with Pregex
Using Anchors and Quantifiers, Grouping and Capturing Matches, Handling Special Characters, and Performance Optimization are essential for efficient string matching with Pregex.
Integrating Pregex with Other Python Libraries
Pregex can be seamlessly integrated with other Python libraries, such as Pandas, Regular Expressions, and NLP libraries, to enhance its functionality and utility in various applications.
Best Practices for String Matching with Pregex
Writing clear and concise patterns, testing and validating patterns, and error handling and exception management are some of the best practices to follow when working with Pregex for string matching.
Also Read: String Data Structure in Python | Complete Case study
Conclusion
In conclusion, Pregex is a valuable tool for string matching in Python, offering a simpler and more intuitive approach than traditional regular expressions. By following the tips and best practices outlined in this article, you can leverage Pregex’s power to match strings in your Python projects efficiently. So, give Pregex a try and streamline your string-matching tasks today!
For more articles on Python, explore our article section today.
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/05/python-string-matching-with-pregex/