Introduction
Python stands out for its simplicity, versatility, and power in the realm of programming languages. The modulo operator (%) holds a special place among its myriad built-in operators, offering a convenient means to calculate remainders and perform cyclic operations. However, despite its apparent simplicity, mastering the modulo operator can be a stumbling block for many Python enthusiasts. In this comprehensive guide, we delve deep into the intricacies of the Python modulo operator. So whether you’re a beginner just starting with Python or an experienced programmer looking to deepen your understanding.
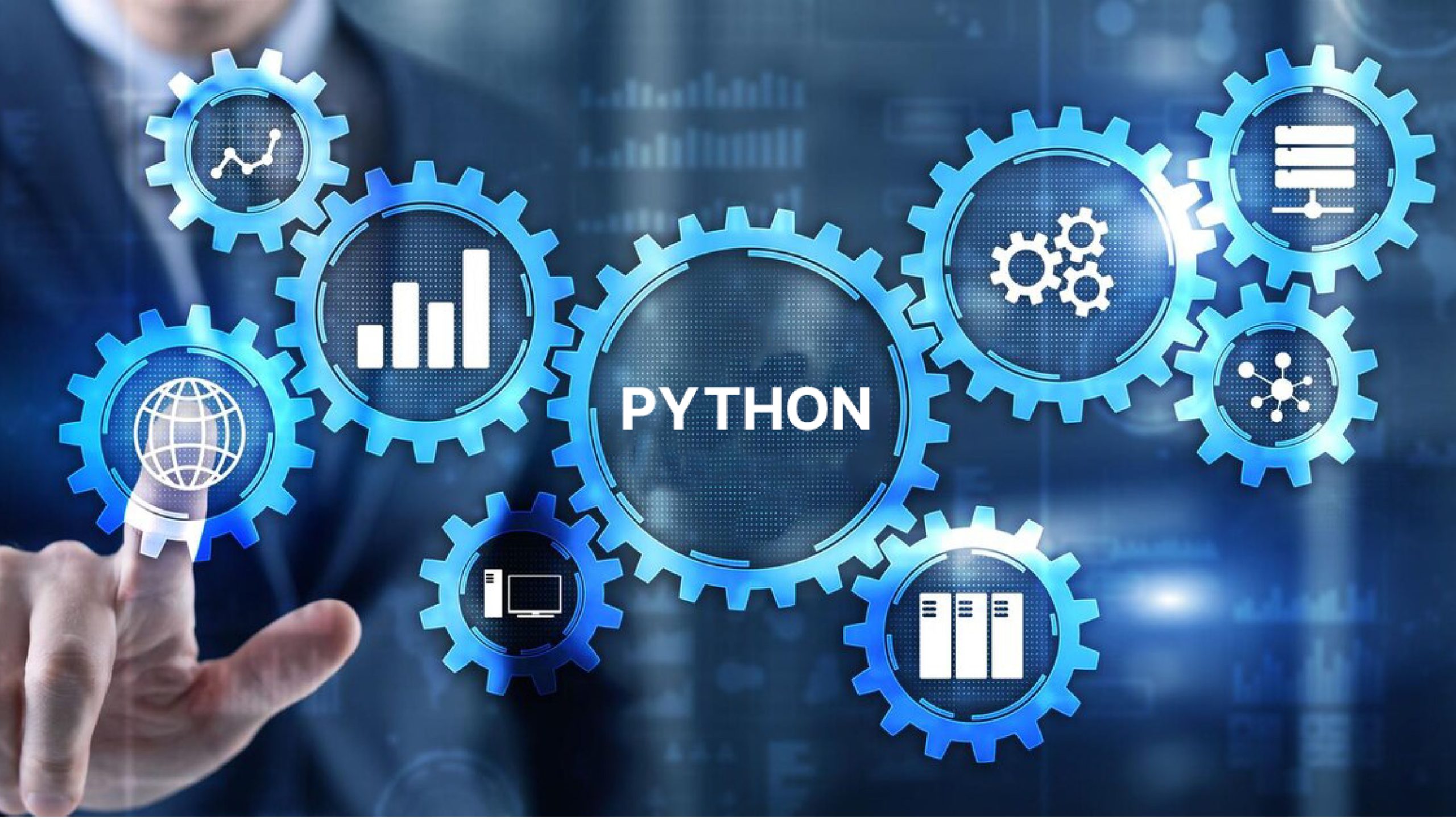
Table of contents
Modulo Operator Basics
What is the Python Modulo Operator?
The Python Modulo Operator, represented by the symbol %, is a mathematical operator that calculates the remainder of a division operation.
Here’s a simple example: 10 % 3 would return 1, because when you divide 10 by 3, you get a quotient of 3 and a remainder of 1.
The Modulo Operator is not just limited to integers. It can also be used with floating-point numbers. For instance, 10.5 % 3 would return 1.5.
One interesting aspect of the Python Modulo Operator is its behavior with negative numbers. If you have a negative number as the dividend, the result will be positive but if the divisor is negative then the remainder will also be negative. For example, -10 % 3 would return 1 but 10 % -3 would be -1.
The Python Modulo Operator is quite versatile and can be used in a variety of real-world scenarios, such as calculating the parity of a number (even or odd), wrapping values within a range, and more.
The Modulo Operator, often symbolized by the % sign, is a mathematical operator that finds the remainder of division between two numbers. It’s a fundamental concept in programming and mathematics with a wide range of applications.
Division: The modulo operation involves two numbers. The first number is divided by the second number. For example, if we have 10 % 3, 10 is divided by 3.
Code:
dividend = 10
divisor = 3
remainder = dividend % divisor
print("The remainder of {} divided by {} is {}.".format(dividend, divisor, remainder))
Output:
The remainder of 10 divided by 3 is 1.
Finding the Remainder: Instead of returning the result of the division, the modulo operation returns the remainder. In our example, 10 % 3 would return 1, because 10 divided by 3 equals 3 with a remainder of 1.
Code:
dividend = 10
divisor = 3
quotient = dividend // divisor
remainder = dividend % divisor
print("The result of {} divided by {} is {} with a remainder of {}.".format(dividend, divisor, quotient, remainder))
Output:
The result of 10 divided by 3 is 3 with a remainder of 1.
Data Types: The modulo operator can use integers and floating-point numbers. For example, 10 % 3 and 10.5 % 3.2 are both valid.
Code:
int_dividend = 10
int_divisor = 3
int_remainder = int_dividend % int_divisor
print("The remainder of {} divided by {} is {}.".format(int_dividend, int_divisor, int_remainder))
Output:
The remainder of 10 divided by 3 is 1.
Code:
float_dividend = 10.5
float_divisor = 3.2
float_remainder = float_dividend % float_divisor
print("The remainder of {} divided by {} is {}.".format(float_dividend, float_divisor, float_remainder))
Output:
The remainder of 10.5 divided by 3.2 is 0.9000000000000004.
Negative Numbers: When dealing with negative numbers, the Python Modulo Operator follows the “floored division” convention. For example, -10 % 3 would return 2, not -1. This is because -10 // 3 equals -4 with a remainder of 2.
Code:
negative_dividend = -10
divisor = 3
remainder = negative_dividend % divisor
print("The remainder of {} divided by {} is {}.".format(negative_dividend, divisor, remainder))
floored_result = negative_dividend // divisor
print("The result of {} divided by {} using floored division is {}.".format(negative_dividend, divisor, floored_result))
Output:
The remainder of -10 divided by 3 is 2.
The result of -10 divided by 3 using floored division is -4.
Zero Division: One important thing to remember is that the divisor (the second number) cannot be zero, as division by zero is undefined in mathematics. If you try to perform a modulo operation with zero as the divisor, Python will raise a ZeroDivisionError.
Code:
dividend = 10
divisor = 3
remainder = dividend % divisor
print("The remainder of {} divided by {} is {}.".format(dividend, divisor, remainder))
Output:
The remainder of 10 divided by 3 is 1.
How Does the Python Modulo Operator Work?
The Python Modulo Operator, denoted by %, works by dividing the number on the left by the number on the right and then returning the remainder of that division.
Let’s break it down with an example. If we have 10 % 3:
- Python first performs the division: 10 ÷ 3. The result of this operation is 3.33 when we carry it out to a few decimal places.
- However, since we’re interested in the remainder, Python looks at how many times 3 can fit into 10 without exceeding 10. In this case, 3 can fit into 10 three times exactly, which gives us 9.
- Finally, Python calculates the difference between the original number (10) and the largest number that is less than 10 and is a multiple of 3 (which is 9). The difference is 1, so 10 % 3 returns 1.
The Python Modulo Operator can also work with floating-point numbers. For example, 10.5 % 3 would perform the division 10.5 ÷ 3, determine that 3 fits into 10.5 three times with a bit left over, and return that bit left over, which in this case is 1.5.
Modulo Operator with Different Numeric Types
Python Modulo Operator with integers
Using the Python Modulo Operator with integers is straightforward. The symbol % represents the operator. Here’s how you can use it:
Choose two integers: The first is the dividend (the number to be divided), and the second is the divisor (the number by which the dividend is divided). For example, let’s choose 10 as the dividend and 3 as the divisor.
Apply the Modulo Operator: You would write this operation as 10 % 3 in Python. This expression tells Python to divide 10 by 3 and return the remainder.
Interpret the result: When you run 10 % 3 in a Python environment, it will return 1. This is because 3 goes into 10 three times, which equals 9, and leaves a remainder of 1.
Code:
dividend = 10
divisor = 3
remainder = dividend % divisor
print("The remainder of {} divided by {} is {}.".format(dividend, divisor, remainder))
Output:
The remainder of 10 divided by 3 is 1.
Python Modulo Operator with floats
The Python Modulo Operator, represented by %, can also be used with floating-point numbers (or floats). Here’s how you can do it:
Choose two floats: The first is the dividend (the number to be divided), and the second is the divisor (the number by which the dividend is divided). For example, let’s choose 10.5 as the dividend and 3.2 as the divisor.
Apply the Modulo Operator: You would write this operation as 10.5 % 3.2 in Python. This expression tells Python to divide 10.5 by 3.2 and return the remainder.
Interpret the result: When you run 10.5 % 3.2 in a Python environment, it will return 0.9. This is because 3.2 goes into 10.5 three times, which equals 9.6, and leaves a remainder of 0.9.
Code:
dividend = 10.5
divisor = 3.2
remainder = dividend % divisor
print("The remainder of {} divided by {} is {}.".format(dividend, divisor, remainder))
Output:
The remainder of 10.5 divided by 3.2 is 0.9.
Python Modulo Operator with a negative number
The Python Modulo Operator, represented by %, behaves a bit differently when used with negative numbers.
Choose two numbers: One or both of these can be negative. For example, let’s choose -10 as the dividend and 3 as the divisor.
Apply the Modulo Operator: You would write this operation as -10 % 3 in Python. This expression tells Python to divide -10 by 3 and return the remainder.
Interpret the result: When you run -10 % 3 in a Python environment, it will return 2. This is because 3 goes into -10 three times, which equals -9, and leaves a remainder of 2.
Code:
dividend = -10
divisor = 3
remainder = dividend % divisor
print("The remainder of {} divided by {} is {}.".format(dividend, divisor, remainder))
Output:
The remainder of -10 divided by 3 is 2.
This might seem counterintuitive at first, but it’s based on Python’s decision to make the result of the modulo operation have the same sign as the divisor. This is known as “floored division”.
How to Override .__mod__() in Python Classes to Use Them with the Modulo Operator?
In Python, you can customize the behavior of operators for user-defined classes by overriding special methods. The .__mod__() method is one such special method that can be overridden to customize the behavior of the modulo operator (%). Here’s how you can do it:
Define a class
First, you need to define a class. For example, let’s create a class named MyNumber.
class MyNumber:
def __init__(self, value):
self.value = value
Override the .__mod__() method
Inside the class, you can define a method named .__mod__(). This method should take one argument besides self, representing the other operand in the modulo operation.
class MyNumber:
def __init__(self, value):
self.value = value
def __mod__(self, other):
return self.value % other.value ** 2
In this example, the .__mod__() method has been overridden to return the remainder of the division of the value of the current object by the square of the value of the other object.
Use the modulo operator with instances of the class
Now, you can create instances of MyNumber and use the modulo operator with them.
# Create two instances of MyNumber
num1 = MyNumber(10)
num2 = MyNumber(3)
# Use the modulo operator with num1 and num2
result = num1 % num2
print("The result of the custom modulo operation is {}.".format(result))
Output:
The result of the custom modulo operation is 1.
Advanced Uses of the Python Modulo Operator
The Python Modulo Operator, represented by %, is not just for finding the remainder of a division operation. It has several advanced uses that can be incredibly useful in your coding journey. Here are a few examples:
Formatting Strings
In Python, the modulo operator can be used for string formatting. For example, you can use it to insert values into a string with placeholders:
name = "Alice"
age = 25
print("Hello, my name is %s and I am %d years old." % (name, age))
Working with Time
The modulo operator can be used to convert seconds into hours, minutes, and seconds, which is particularly useful when working with time data:
total_seconds = 3661
hours = total_seconds // 3600
remaining_minutes = (total_seconds % 3600) // 60
remaining_seconds = (total_seconds % 3600) % 60
print("%d hours, %d minutes, and %d seconds" % (hours, remaining_minutes, remaining_seconds))
Creating Circular Lists
The modulo operator can be used to create circular lists, which are lists that wrap around at the end. This is useful in a variety of scenarios, such as game development or data analysis:
items = ['a', 'b', 'c', 'd', 'e']
for i in range(10):
print(items[i % len(items)])
Generating Alternating Patterns in Data Visualization
You can use the modulo operator to cycle through a list of colors or line styles when plotting multiple lines on a single graph. This ensures that each line has a distinct style, improving the readability of the graph.
import matplotlib.pyplot as plt
import numpy as np
colors = ['b', 'g', 'r', 'c', 'm', 'y', 'k']
x = np.linspace(0, 10, 100)
y = [np.sin(x + i) for i in range(7)]
for i in range(7):
plt.plot(x, y[i], color=colors[i % len(colors)])
plt.show()
Creating a Simple Hash Function
The modulo operator can be used to create a simple hash function, which maps data of arbitrary size to fixed-size values. This is useful in many areas of computer science, including data retrieval and cryptography.
def simple_hash(input_string, table_size):
sum = 0
for pos in range(len(input_string)):
sum = sum + ord(input_string[pos])
return sum % table_size
print(simple_hash("Hello, World!", 10))
Implementing a Circular Buffer
A circular buffer is a data structure that uses a single, fixed-size buffer as if connected end-to-end. This structure lends itself to buffering data streams. The modulo operator can calculate the index in the buffer to which the next value (or the next several values) will be written.
class CircularBuffer:
def __init__(self, size):
self.buffer = [None] * size
self.size = size
self.index = 0
def add(self, value):
self.buffer[self.index] = value
self.index = (self.index + 1) % self.size
def __str__(self):
return str(self.buffer)
buffer = CircularBuffer(5)
for i in range(10):
buffer.add(i)
print(buffer)
How to Use the Python Modulo Operator to Solve Real-World Problems?
The Python Modulo Operator, represented by %, is a versatile tool that can be used to solve a variety of real-world problems. Here are a few examples:
Determining if a number is even or odd: In Python, you can use the modulo operator to quickly check if a number is even or odd. If number % 2 equals 0, the number is even. If it equals 1, the number is odd.
number = 7
if number % 2 == 0:
print("{} is even.".format(number))
else:
print("{} is odd.".format(number))
Creating a wrapping effect: The modulo operator can be used to create a wrapping effect, which is useful in many areas such as game development. For example, if you have a list of 5 elements and you want to get the next element in a circular manner, you can use (index + 1) % 5.
elements = ['a', 'b', 'c', 'd', 'e']
index = 4
next_index = (index + 1) % len(elements)
print("The next element after {} is {}.".format(elements[index], elements[next_index]))
Converting seconds to hours, minutes, and seconds: If you have a large number of seconds, you can use the modulo operator to convert it into hours, minutes, and seconds.
seconds = 3661
hours = seconds // 3600
minutes = (seconds % 3600) // 60
remaining_seconds = (seconds % 3600) % 60
print("{} seconds is equal to {} hours, {} minutes, and {} seconds.".format(seconds, hours, minutes, remaining_seconds))
Calculating Leap Years: The modulo operator can be used to determine if a year is a leap year. A year is a leap year if it is divisible by 4, but not divisible by 100, unless it is also divisible by 400.
year = 2000
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
print("{} is a leap year.".format(year))
else:
print("{} is not a leap year.".format(year))
Creating Alternating Patterns: The modulo operator can be used to create alternating patterns, which can be useful in a variety of scenarios, such as alternating row colors in a table for better readability.
for i in range(10):
if i % 2 == 0:
print("This is an even row.")
else:
print("This is an odd row.")
Ensuring Limited Input Range: The modulo operator can be used to ensure that an input number falls within a certain range. For example, if you’re building a clock and you want to ensure that the entered hour falls within the 0-23 range, you can use the modulo operator.
hour = 25
hour = hour % 24
print("The hour on a 24-hour clock is {}.".format(hour))
Common Errors and How to Handle Them
You might encounter a few common errors when working with the Python Modulo Operator. Here’s how to handle them:
ZeroDivisionError
This error occurs when you try to divide by zero. In the context of the modulo operation, it happens when the divisor is zero. To handle this error, you can use a try-except block:
try:
result = 10 % 0
except ZeroDivisionError:
print("Error: Division by zero is not allowed.")
TypeError
This error occurs when you try to use the modulo operator with incompatible types, such as a string and an integer. To handle this error, you can ensure that both operands are numbers:
try:
result = "10" % 3
except TypeError:
print("Error: Modulo operation requires numbers.")
AttributeError
If you’re working with custom classes and you haven’t implemented the .__mod__() method, you might encounter this error when trying to use the modulo operator. To handle this error, you can implement the .__mod__() method in your class:
class MyClass:
def __init__(self, value):
self.value = value
def __mod__(self, other):
return self.value % other.value
try:
result = MyClass(10) % MyClass(3)
except AttributeError:
print("Error: Modulo operation not supported for this class.")
Sure, here are three more common errors and how to handle them when working with the Python Modulo Operator:
Floating Point Precision Errors
When working with floating-point numbers, you might encounter precision errors due to the way these numbers are represented in memory. To handle this, you can use the round() function to limit the number of decimal places:
result = 10.2 % 3.1
print("The result is {:.2f}.".format(result))
Modulo with Complex Numbers
The modulo operation is not defined for complex numbers in Python. If you try to use the modulo operator with complex numbers, you’ll get a TypeError. To handle this, you can check if the operands are complex before performing the operation:
try:
result = (1+2j) % (3+4j)
except TypeError:
print("Error: Modulo operation is not supported for complex numbers.")
Modulo with NoneType
If one of the operands is None, you’ll get a TypeError. To handle this, you can check if the operands are None before performing the operation:
try:
result = None % 3
except TypeError:
print("Error: Modulo operation requires numbers, not NoneType.")
Conclusion
The Python Modulo Operator is a versatile tool that can be used in various ways, from basic arithmetic to advanced programming concepts. We’ve explored its usage with different numeric types, how to override the .__mod__() method in Python classes, and its real-world applications. We’ve also delved into advanced uses and common errors. Understanding the Python Modulo Operator is key to mastering Python arithmetic and can open up new possibilities in your coding journey.
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/02/python-modulo-operator/