Introduction
The division operator is a cornerstone in Python programming, facilitating mathematical computations and maneuvers. Mastering its functionality holds paramount importance in crafting proficient and precise code. This extensive manual ventures into the depths of the division operator in Python, encompassing its diverse manifestations and utilities. We will scrutinize integer division, float division, floor division, and truncating division, furnishing lucid elucidations and illustrative instances for each variant. Upon concluding this discourse, you will fully understand the optimal utilization of these division operators, emboldening your adeptness in navigating mathematical intricacies within your Python endeavors.
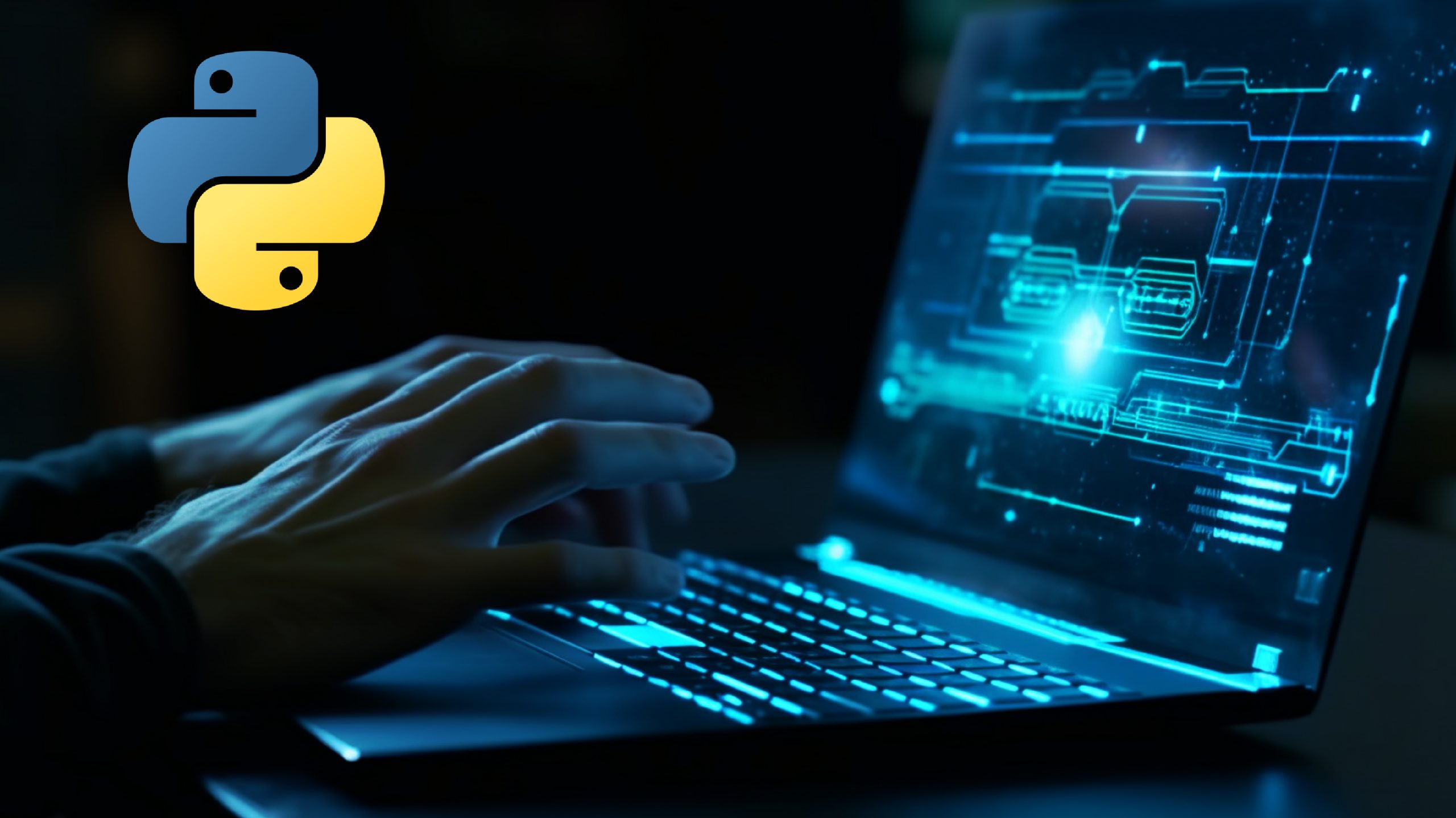
Table of contents
Understanding the Division Operator in Python
The division operator in Python can be used with integers and floating-point numbers. When you divide two integers using the division operator, the result will always be a floating-point number.
Code:
a = 10
b = 3
result = a / b
print(result)
Output:
3.3333333333333335
In this code, we divide the integer 10 by the integer 3. The result is a floating-point number: 3.3333333333335.
If you want to perform integer division and get an integer result, you can use the double forward slash “//” operator. This will return the floor division of two numbers, discarding any remainder.
Code:
a = 10
b = 3
result = a // b
print(result)
Output:
3
There will be three outcomes in the given code excerpt since the division’s fractional component gets truncated. Grasping the subtleties of Python’s division operator enhances your ability to craft efficient and precise code for mathematical tasks. Delve into various scenarios and data types to master this foundational Python concept.
Types of Division Operators in Python
There are several types of division operators that you can use in Python. Let’s dive into each one:
Integer Division
Integer division in Python is denoted by the double forward slash (//) operator. It returns the quotient of the division operation, discarding any remainder.
Code:
result = 10 // 3
print(result)
Output:
3
Float Division
Float division in Python is denoted by the single forward slash (/) operator. It returns the division operation’s quotient as a floating-point number.
Code:
result = 10 / 3
print(result)
Output:
3.3333333333333335
Floor Division
Floor division in Python is similar to integer division but always rounds down to the nearest integer. It is denoted by the double forward slash (//) operator.
Code:
result = 10.0 // 3
print(result)
Output:
3.0
Truncating Division
Truncating division in Python is similar to floor division but always rounds towards zero. The percent (%) operator denotes it.
Code:
result = 10.0 % 3
print(result)
Output:
1.0
Each type of division operator in Python has its use case and can be handy in different situations. Experiment with these operators in your code to see how they work and which one best suits your needs.
Division Operator Techniques and Examples
Regarding division in Python, there are various techniques and scenarios to consider. Let’s explore common examples to understand how division operators work in different situations.
Division with Integers
When you divide two integers in Python, the result will always be a float if there is a remainder. For example:
Code:
a = 10
b = 3
result = a / b
print(result)
Output:
3.3333333333333335
Division with Floats
Dividing floats in Python is straightforward and follows the same rules as dividing integers. Here’s an example:
Code:
x = 7.5
y = 2.5
result = x / y
print(result)
Output:
3.0
Division with Negative Numbers
When dealing with negative numbers, the division operator behaves as expected. For instance:
Code:
m = -8
n = 2
result = m / n
print(result)
Output:
-4.0
Division with Zero
Dividing by zero in Python will raise a ZeroDivisionError. It is not possible to divide any number by zero in Python. Here’s an example:
Code:
p = 10
q = 0
Try:
result = p / q
print(result)
except ZeroDivisionError:
print("Division by zero is not allowed!")
Output:
Division by zero is not allowed!
By understanding these division operator techniques and examples, you can effectively utilize the division operator in Python for various mathematical operations.
Conclusion
Acquiring proficiency in utilizing the division operator within Python is a pivotal asset that enriches your programming prowess. In this discourse, we’ve delved into the diverse facets of division operators, elucidating their practical implications and providing strategies for navigating through assorted scenarios. These encompass scenarios like division involving integers, floats, negative values, and even the rare case of division by zero. Empowered with this newfound understanding, you can seamlessly integrate the appropriate division operator into your codebase, ensuring precision and efficiency in mathematical computations. Consistent practice and exploration of the division operator will cement its status as an indispensable instrument within your programming arsenal.
To strengthen your Python programming skills, check out this free course.
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/04/division-operator-in-python/