Introduction
In this article, you’ll discover an in-depth exploration of the sum()
function in Python, a crucial built-in utility for aggregating numbers across various iterable types. We begin by explaining the fundamental syntax and parameters of the sum() function, followed by detailed examples showcasing its application to lists, dictionaries, sets, and tuples. Additionally, you’ll learn about error handling to prevent common pitfalls and see practical scenarios, such as calculating averages, where sum()
proves invaluable. This guide aims to enhance your understanding and usage of sum()
in everyday programming tasks, making your code more efficient and readable.
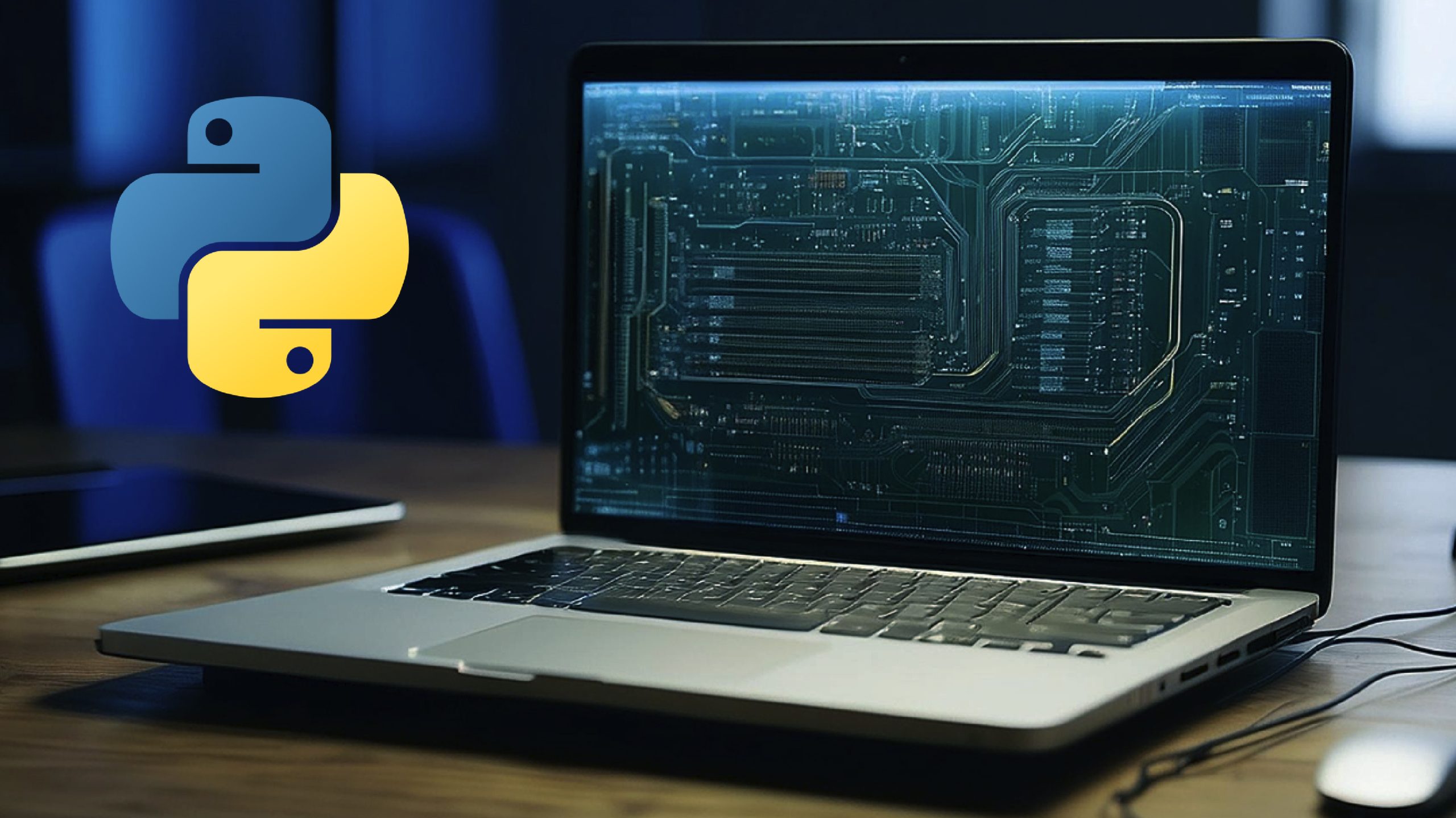
Table of contents
What is sum() Function?
You may compute the sum of the numbers in an iterable using Python’s robust built-in sum() function. In jobs involving data analysis and processing, this function is commonly utilized. Let us understand the syntax of sum() function.
Syntax of sum()
Function
The sum() function syntax is straightforward:
sum(iterable, start)
- iterable: Any iterable holding numerical values, such as a list, tuple, set, dictionary, etc., can be this.
- start: This optional argument adds a specified value to the sum of the iterable’s values. In the absence of one, it defaults to 0.
Simplified Syntax Variations
sum(a)
: Calculates the total number in the list a, with 0 as the initial value by default.sum(a, start)
: Computes the sum of the numbers in the lista
and adds thestart
value to the result.
Basic Usage
Let’s start with a basic example where we sum a list of numbers to better understand how the sum() function functions. This basic example will illustrate the core functionality of sum() in a straightforward manner.
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(total) # Output: 15
Using the start Parameter
The start
parameter allows you to add a value to the sum of the iterable. Here’s how you can use it:
numbers = [1, 2, 3, 4, 5]
total = sum(numbers, 10)
print(total) # Output: 25
Examples of Using sum()
Let us now explore some examples of using sum().
Summing Numbers in a List
Let’s start with a basic example of summing numbers in a list.
expenses = [200, 150, 50, 300]
total_expenses = sum(expenses)
print(total_expenses) # Output: 700
Summing Values in a Dictionary
You can sum the values of a dictionary using the values()
method.
my_dict = {'x': 21, 'y': 22, 'z': 23}
total = sum(my_dict.values())
print(total) # Output: 66
Summing Elements in a Set
Sets, like lists, can be summed directly.
unique_numbers = {12, 14, 15}
total_unique = sum(unique_numbers)
print(total_unique) # Output: 39
Summing Elements in a Tuple
Tuples, similar to lists, can also be summed.
scores = (90, 85, 88, 92)
total_scores = sum(scores)
print(total_scores) # Output: 355
Summing Numbers Using a For Loop
Although the sum()
function is convenient, you can also sum numbers manually using a for loop.
numbers = [100, 200, 300, 400, 500]
# Initialize the sum to 0
total = 0
# Iterate through the list and add each number to the total
for num in numbers:
total += num
print("The sum of the numbers is:", total) # Output: 1500
Summing with a Generator Expression
Generator expressions can be used to sum a series of numbers generated on the fly:
total = sum(i for i in range(1, 6))
print(total) # Output: 15
Summing the Elements of a List of Lists
Sometimes, you may have a list of lists and want to sum the elements within each list. Here’s how you can do it:
list_of_lists = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
total = sum(sum(inner_list) for inner_list in list_of_lists)
print(total) # Output: 45
Advanced Applications
Let us explore some advanced applications of sum() function.
Summing Non-Numeric Values
While sum() is primarily used for numeric values, you can use it in conjunction with other functions to sum non-numeric values. For example, summing the lengths of strings in a list:
words = ["apple", "banana", "cherry"]
total_length = sum(len(word) for word in words)
print(total_length) # Output: 16
Summing with Conditional Logic
You can incorporate conditional logic within a generator expression to sum values that meet certain criteria:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
total_even = sum(n for n in numbers if n % 2 == 0)
print(total_even) # Output: 30
Error Handling
The sum() function raises a TypeError if the iterable contains non-numeric values.
array1 = ["apple"]
try:
# Attempt to sum the list of strings
total = sum(array1)
except TypeError as e:
print(e) # Output: unsupported operand type(s) for +: 'int' and 'str'
Practical Application
Let us now look into the practical applications of sum() function.
Summing the Ages of a List of People
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
people = [Person("Alice", 30), Person("Bob", 25), Person("Charlie", 35)]
# Calculate the total sum of ages
total_age = sum(person.age for person in people)
print(total_age) # Output: 90
Financial Calculations
Sum() can be used for financial calculations, such as computing the total expenses or income over a period.
Calculating Total Expenses:
expenses = {
'rent': 1200,
'groceries': 300,
'utilities': 150,
'entertainment': 100
}
# Calculate the total sum of expenses
total_expenses = sum(expenses.values())
print(total_expenses) # Output: 1750
Calculating Yearly Income:
monthly_income = [2500, 2700, 3000, 3200, 2900, 3100, 2800, 2600, 3300, 3500, 3400, 3600]
# Calculate the total sum of yearly income
yearly_income = sum(monthly_income)
print(yearly_income) # Output: 37600
Conclusion
For adding up the numbers in different iterables, Python’s sum() method provides a flexible and effective tool. It is a vital tool for any programmer’s toolset because of its simplicity of usage and Python’s strong error handling. The sum() function makes aggregation simpler and enables straightforward, short code, regardless of whether one is working with lists, tuples, sets, or dictionaries.
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/05/sum-function-in-python/