Introduction
Navigating through JSON data in Python opens doors to seamless data manipulation and analysis. JSON, or JavaScript Object Notation, is a lightweight data exchange format widely employed online. This guide discusses the significance of reading JSON files within Python’s versatile ecosystem. Discover various methods, from leveraging the JSON module to utilizing Pandas and best practices ensuring efficient data handling. Unravel the potential of JSON data manipulation in Python for endless possibilities in coding endeavors.
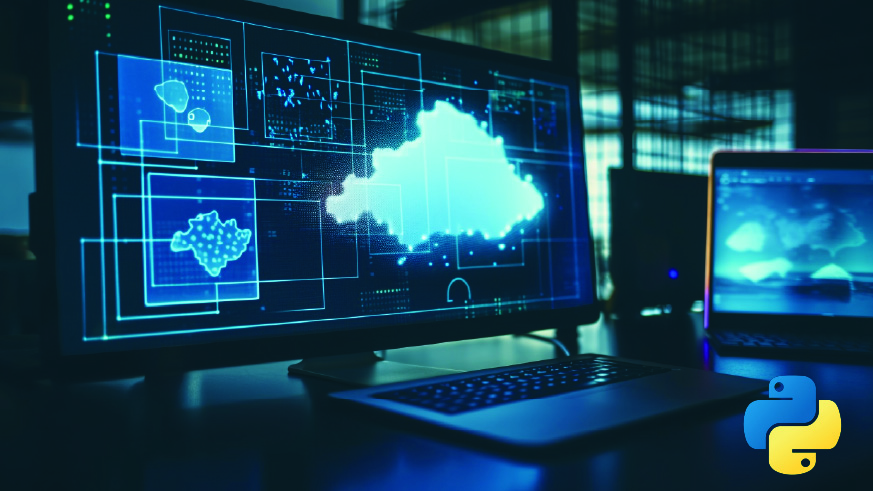
Table of contents
Why Read JSON Files in Python?
Understanding the significance of reading JSON files in Python boils down to the language’s adaptability and the ubiquity of JSON as a data format on the web. Python’s inherent versatility, coupled with its rich ecosystem of libraries and tools, facilitates seamless manipulation and integration of JSON data. This proficiency equips developers with the means to efficiently access, extract, and modify information stored in JSON files, streamlining their workflow and enhancing productivity.
Methods to Read JSON Files in Python
There are several methods to read, each offering advantages and use cases.
Using the json Module
The json module in Python provides functions for encoding and decoding JSON data. It allows you to read JSON files and convert them into Python objects effortlessly.
import json
# Read JSON file
with open('data.json') as f:
data = json.load(f)
print(data)
Using the Pandas Library
Pandas, a popular data manipulation library in Python, also supports reading JSON files. It offers additional functionalities for data analysis and manipulation.
import pandas as pd
# Read JSON file
data = pd.read_json('data.json')
print(data)
Using the json.loads() Method
The json.loads() method is used to parse a JSON string and convert it into a Python dictionary.
import json
# JSON string
json_str = '{"name": "John", "age": 30}'
data = json.loads(json_str)
print(data)
Output: {‘name’: ‘John’, ‘age’: 30}
Using the json.dumps() Method
The json.dumps() method is used to serialize a Python object into a JSON formatted string.
import json
# Python object
data = {'name': 'John', 'age': 30}
json_str = json.dumps(data)
print(json_str)
Best Practices for Reading JSON Files in Python
To ensure smooth reading of JSON files in Python, follow these best practices:
- Validating JSON Data: To avoid parsing errors, validate the JSON data before reading it.
- Handling Nested JSON Structures: Handle nested JSON structures by accessing the data using appropriate keys.
- Error Handling and Exception Handling: To manage unexpected issues, implement error handling and exception handling.
- Performance Optimization Techniques: Use performance optimization techniques like caching to improve the efficiency of reading JSON files.
Working with JSON Data in Python
Once you have read the JSON data in Python, you can perform various operations on it.
Accessing JSON Data
Access specific data elements in the JSON file by navigating through the keys.
# Accessing JSON data
print(data['name'])
Modifying JSON Data
Modify the JSON data by updating existing values or adding new key-value pairs.
# Modifying JSON data
data['age'] = 35
print(data)
Extract specific information from the JSON data based on your requirements.
# Extracting specific information
for item in data['items']:
print(item['name'])
Also read: Python json.loads() and json.dump() methods
Conclusion
Reading JSON files in Python is a fundamental skill for any developer working with data. Using the various methods and best practices outlined in this guide, you can efficiently read, manipulate, and extract valuable information from JSON files in Python.
Remember to validate the JSON data, handle errors gracefully, and optimize performance for a seamless experience. Start exploring the world of JSON data in Python and unlock endless possibilities for data manipulation and analysis.
Are you looking for an online course on Python? If yes, explore – Learn Python for Data Science.
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/03/heres-how-you-can-read-json-files-in-python/