Introduction
In the digital realm, effective data management is paramount for any software application, and Python, with its simplicity and robust capabilities, emerges as a preferred language for developers. Whether you’re a novice or a seasoned coder, mastering file handling in Python is a foundational skill that promises versatility. This blog delves into the essentials of file handling in Python, ensuring you can navigate your data with confidence and ease.
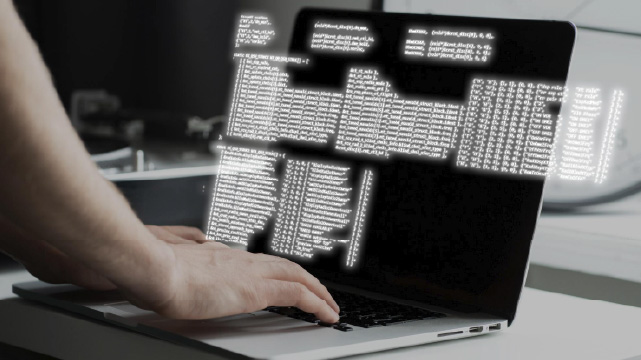
Table of contents
Open Function
To engage with a file, it must first be opened. Python’s built-in open() function plays a pivotal role, returning a file object and serving as the gateway to your file’s content. The function requires the file path and the desired mode, such as ‘r’ for reading, ‘w’ for writing, ‘a’ for appending, or ‘r+’ for both reading and writing.
You can also read more here to know about list files in a directory using python.
Reading Files
Once a file is open in read mode, Python offers methods like read(), readline(), and readlines() to suit various reading requirements. The read() method outputs the entire content at once, while readline() fetches one line at a time. Alternatively, readlines() presents the entire file content as a list of lines.
Code Example
# File path
file_path = 'example.txt'
# Open the file in 'r' mode (read mode)
file = open(file_path, 'r')
# Read the content of the file
content = file.read()
Writing Files
Writing to a file in Python is as straightforward as reading. With the file opened in write or append mode, the write() or writelines() methods come into play. The write() method takes a string and engraves it onto the file, while writelines() processes a list of strings, inscribing each as a separate line. It’s essential to note that opening a file in write mode erases existing content, so handle with care!
Usage of ‘with’ Statement
Python’s ‘with’ statement streamlines file handling by automating the opening and closing process. It acts as a personal assistant, opening the file, allowing you to perform operations, and then dutifully closing it once the task is complete. This not only enhances code cleanliness but also guards against potential file mishaps.
# File path
file_path = 'example.txt'
# Open the file in 'r' mode (read mode) using 'with'
with open(file_path, 'r') as file:
# Read the content of the file
content = file.read()
print("File content:")
print(content)
# File is automatically closed outside the 'with' block
Handling File Paths
Efficient navigation of file paths is pivotal in file handling. Python’s os.path module, acting as a GPS, provides functions like os.path.join() to construct paths in a system-agnostic manner. This ensures code portability and minimizes the risk of path-related errors.
Error Handling: Safeguarding Your File Operations
In the file handling domain, errors are akin to road potholes. Python’s try-except blocks serve as your suspension system, absorbing shocks from FileNotFoundError, IOError, and other exceptions. Wrapping file operations in these blocks ensures graceful handling of unexpected challenges.
# File path
file_path = 'nonexistent_file.txt'
try:
# Open the file in 'r' mode (read mode)
with open(file_path, 'r') as file:
# Read the content of the file
content = file.read()
print("File content:")
print(content)
except FileNotFoundError:
print(f"The file '{file_path}' was not found.")
except IOError as e:
print(f"An I/O error occurred: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Best Practices: Writing Future-Proof Code
To craft code that endures, adhere to best practices such as closing files after use, robust exception handling, and employing context managers. These practices not only enhance code resilience and maintainability but also garner respect from peers and future-proof your work.
Conclusion
Becoming adept at file handling in Python empowers you with the ability to manage data effectively. By mastering the techniques of opening, reading, writing, and navigating files, you equip yourself with versatile tools for diverse coding endeavors. Utilize context managers for cleaner code, handle exceptions to prevent crashes, and follow best practices for code robustness and maintainability.
Become a python expert with our FREE python course.
Frequently Asked Questions:
A. File handling in Python is crucial for managing data within software applications. It allows developers to read from and write to files, enabling the storage and retrieval of information, which is fundamental to most programming tasks.
A. The ‘with’ statement simplifies file handling by automatically managing the file’s opening and closing. It acts as a context manager, ensuring that the file is properly closed even if an exception occurs. This enhances code readability and reduces the risk of resource leaks.
A. The ‘open()’ function in Python is used to open files and returns a file object. It is crucial for file handling as it provides access to a file’s content, serving as the gateway to reading or writing data. The function takes the file path and mode as parameters.
Related
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/01/file-handeling-in-python/